Sticky
Bootstrap 5 Sticky
Sticky is a directive which allows elements to be locked in a particular area of the page. It is often used in navigation menus.
Note: Read the API tab to find all available options and advanced customization
Basic example
To start use sticky just add a v-mdb-sticky
directive to the element you want to
pin
<template>
<div style="min-height: 500px" class="text-center">
<MDBBtn
tag="a"
outline="primary"
color="white"
v-mdb-sticky="{
boundary: true
}"
id="sticky"
href="https://mdbootstrap.com/docs/vue/getting-started/installation/"
>Download MDB
</MDBBtn>
</div>
</template>
<script>
import { MDBBtn, mdbSticky } from 'mdb-vue-ui-kit';
export default {
components: {
MDBBtn,
},
directives: {
mdbSticky,
},
setup() {
return {};
},
};
</script>
Sticky bottom
You can pin element to bottom by adding
v-mdb-sticky="{ position: 'bottom' }"
<template>
<div style="min-height: 500px" class="text-center">
<img
v-mdb-sticky="{
position: 'bottom',
boundary: true,
direction: 'both'
}"
id="MDB-logo-1"
src="https://mdbootstrap.com/wp-content/uploads/2018/06/logo-mdb-jquery-small.webp"
alt="MDB Logo"
/>
</div>
</template>
<script>
import { mdbSticky } from 'mdb-vue-ui-kit';
export default {
directives: {
mdbSticky,
},
setup() {
return {};
},
};
</script>
Boundary
Set v-mdb-sticky="{ boundary: true }"
so that sticky only works inside the parent
element. Remember to set the correct parent height.
<template>
<div style="min-height: 500px" class="text-center">
<img
v-mdb-sticky="{
boundary: true
}"
id="MDB-logo-2"
src="https://mdbootstrap.com/wp-content/uploads/2018/06/logo-mdb-jquery-small.webp"
alt="MDB Logo"
/>
</div>
</template>
<script>
import { mdbSticky } from 'mdb-vue-ui-kit';
export default {
directives: { mdbSticky },
setup() {
return {}
}
};
</script>
Outer element as a boundary
You can specify an element selector to be the sticky boundary.
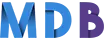
<template>
<div
style="min-height: 500px"
class="d-flex flex-column justify-content-center text-center"
>
<div>
<img
v-mdb-sticky="{
boundary: '#sticky-stop',
direction: 'both'
}"
id="MDB-logo-3"
src="https://mdbootstrap.com/wp-content/uploads/2018/06/logo-mdb-jquery-small.webp"
alt="MDB Logo"
/>
</div>
<div id="sticky-stop" class="mt-auto" style="height: 5rem">Stop here</div>
</div>
</template>
<script>
import { mdbSticky } from 'mdb-vue-ui-kit';
export default {
directives: {
mdbSticky,
},
setup() {
return {};
},
};
</script>
Direction
Default direction of sticky component is down
. You can change it by setting
v-mdb-sticky="{ direction: 'up' }"
or
v-mdb-sticky="{ direction: 'both' }"
<template>
<div style="min-height: 500px" class="text-center">
<MDBBtn
tag="a"
color="primary"
v-mdb-sticky="{
boundary: true,
direction: 'up'
}"
>
Up
</MDBBtn>
<MDBBtn
tag="a"
color="primary"
v-mdb-sticky="{
boundary: true,
direction: 'down'
}"
>
Down
</MDBBtn>
<MDBBtn
tag="a"
color="primary"
v-mdb-sticky="{
boundary: true,
direction: 'both'
}"
>
Both
</MDBBtn>
</div>
</template>
<script>
import { MDBBtn, mdbSticky } from 'mdb-vue-ui-kit';
export default {
components: {
MDBBtn,
},
directives: {
mdbSticky,
},
setup() {
return {};
},
};
</script>
Animation
You can add an animation that will run when the sticky starts and when the sticky element is
hidden. just specify the css class of the animation using the
v-mdb-sticky="{ animationSticky: 'animation-name' }"
and
v-mdb-sticky="{ animationUnsticky: 'animation-name' }"
.
Remember that not every animation will be appropriate. We suggest using the animations used in the example below.
<template>
<div style="min-height: 500px" class="text-center">
<img
v-mdb-sticky="{
boundary: true,
animationSticky: 'slide-in-down',
animationUnsticky: 'slide-up',
delay: '30'
}"
id="MDB-logo-4"
src="https://mdbootstrap.com/wp-content/uploads/2018/06/logo-mdb-jquery-small.webp"
alt="MDB Logo"
/>
</div>
</template>
<script>
import { mdbSticky } from 'mdb-vue-ui-kit';
export default {
directives: {
mdbSticky,
},
setup() {
return {};
},
};
</script>
Sticky - API
Import
<script>
import {
mdbSticky
} from 'mdb-vue-ui-kit';
</script>
Properties
Property | Type | Default | Description |
---|---|---|---|
animationSticky
|
String | '' |
Set sticky animation |
animationUnsticky
|
String | '' |
Set unsticky animation |
boundary
|
Boolean | false |
set to true to stop sticky on the end of the parent |
delay
|
Number | 0 |
Set the number of pixels beyond which the item will be pinned |
direction
|
String | 'down' |
Set the scrolling direction for which the element is to be stikcky |
media
|
Number | 0 |
Set the minimum width in pixels for which the sticky should work |
offset
|
Number | 0 |
Set the distance from the top edge of the element for which the element is sticky |
position
|
String | 'top' |
Set the edge of the screen the item should stick to |