Touch
React Bootstrap 5 Touch
This component allows you to improve the user experience on mobile touch screens by calling methods on the following custom events: pinch, swipe, tap, press, pan and rotate.
Note: Read the API tab to find all available options and advanced customization
Note: This method is intended only for users of mobile touch screens, so it won't work for the mouse or keyboard events.
Press
Press calls the chosen method when the touch event on the element lasts longer than 250 milliseconds.
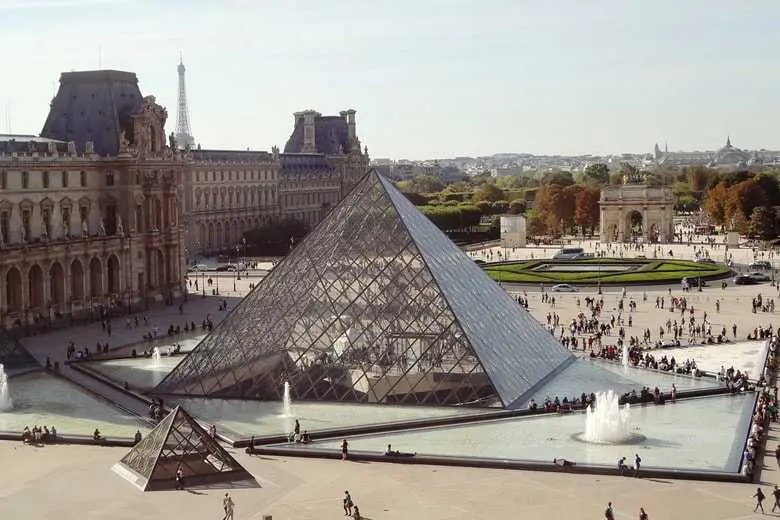
Hold the button to remove the mask from the image
import React, { useState } from 'react';
import { MDBTouch, MDBBtn } from 'mdb-react-ui-kit';
export default function App() {
const [pressColor, setPressColor] = useState('rgba(0, 0, 0, 0.6)');
const handlePress = () => {
setPressColor('rgba(0, 0, 0, 0)');
};
return (
<div>
<div className='bg-image'>
<img src='https://mdbootstrap.com/img/new/standard/city/053.webp' className='img-fluid' />
<div className='mask' style={{ backgroundColor: pressColor }}>
<div className='d-flex justify-content-center align-items-center h-100'>
<p className='text-white mb-0'>Hold the button to remove the mask from the image</p>
</div>
</div>
</div>
<div className='my-3'>
<MDBTouch tag={MDBBtn} className='my-3' type='press' onPress={handlePress}>
{' '}
Tap & hold to show image
</MDBTouch>
</div>
</div>
);
}
Press duration
Touch event press with custom duration.
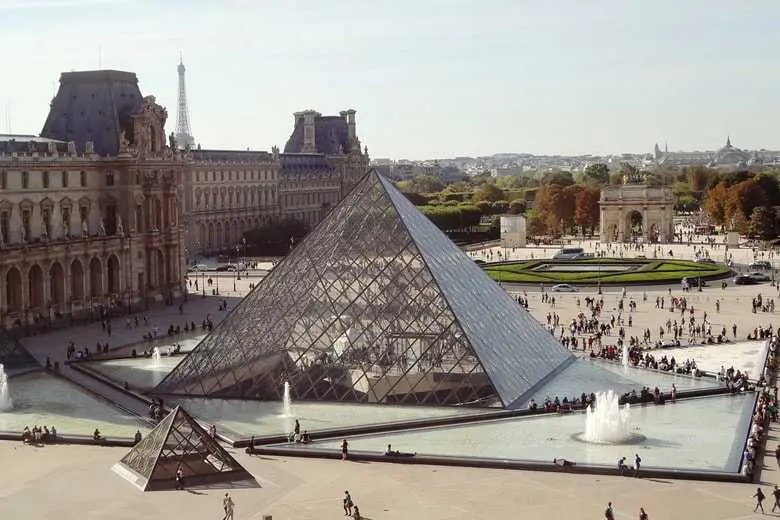
Hold the button to remove the mask from the image with 5 seconds
import React, { useState } from 'react';
import { MDBTouch, MDBBtn } from 'mdb-react-ui-kit';
export default function App() {
const [longPressColor, setLongPressColor] = useState('rgba(0, 0, 0, 0.6)');
const handleLongPress = () => {
setLongPressColor('rgba(0, 0, 0, 0)');
};
return (
<div>
<div className='bg-image'>
<img src='https://mdbootstrap.com/img/new/standard/city/053.webp' className='img-fluid' />
<div className='mask' style={{ backgroundColor: longPressColor }}>
<div className='d-flex justify-content-center align-items-center h-100'>
<p className='text-white mb-0'>Hold the button to remove the mask from the image with 5s</p>
</div>
</div>
</div>
<div className='my-3'>
<MDBTouch tag={MDBBtn} time={5000} className='my-3' type='press' onPress={handleLongPress}>
{' '}
Tap & hold to show image
</MDBTouch>
</div>
</div>
);
}
Tap
The callback on tap event is called with an object containing origin field - the x and y coordinates of the user's touch.
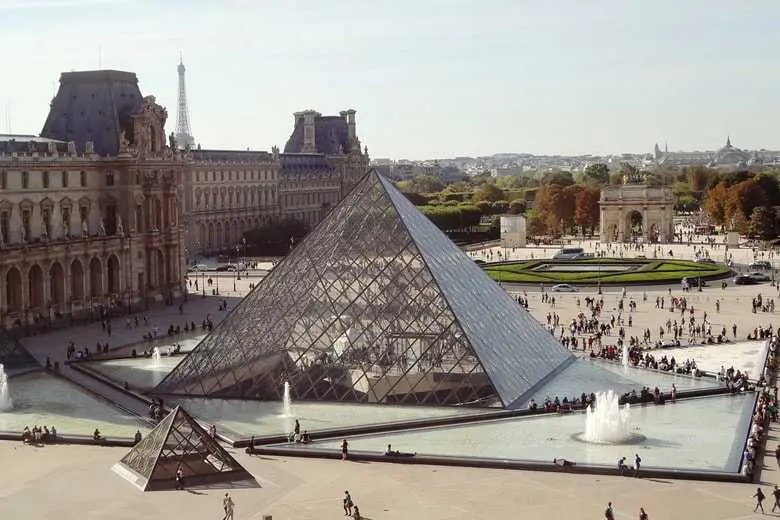
Tap button to change a color
import React, { useState } from 'react';
import { MDBTouch, MDBBtn } from 'mdb-react-ui-kit';
export default function App() {
const [tapColor, setTapColor] = useState('rgba(0, 0, 0, 0.6)');
const colorGen = () => {
const randomNum = () => {
return Math.floor(Math.random() * 255) + 1;
};
return `rgba(${randomNum()},${randomNum()},${randomNum()},.4)`;
};
const handleTap = () => {
setTapColor(colorGen());
};
return (
<div>
<div className='bg-image'>
<img src='https://mdbootstrap.com/img/new/standard/city/053.webp' className='img-fluid' />
<div className='mask' style={{ backgroundColor: tapColor }}>
<div className='d-flex justify-content-center align-items-center h-100'>
<p className='text-white mb-0'>Tap button to change a color</p>
</div>
</div>
</div>
<div className='my-3'>
<MDBTouch duration={2000} tag={MDBBtn} className='my-3' type='tap' onTap={handleTap}>
{' '}
Tap to change a color
</MDBTouch>
</div>
</div>
);
}
Double Tap
Set default taps to touch event.
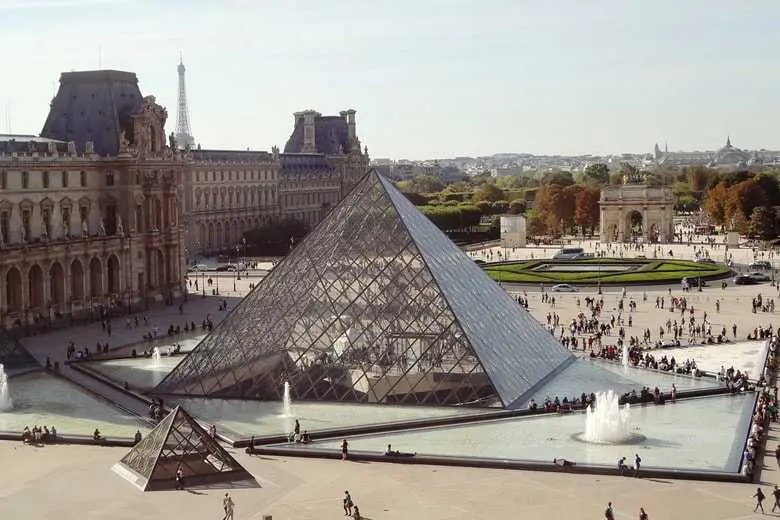
Change background color with 2 taps
import React, { useState } from 'react';
import { MDBTouch, MDBBtn } from 'mdb-react-ui-kit';
export default function App() {
const [doubleTapColor, setDoubleTapColor] = useState('rgba(0, 0, 0, 0.6)');
const colorGen = () => {
const randomNum = () => {
return Math.floor(Math.random() * 255) + 1;
};
return `rgba(${randomNum()},${randomNum()},${randomNum()},.4)`;
};
const handleDoubleTap = () => {
setDoubleTapColor(colorGen());
};
return (
<div>
<div className='bg-image'>
<img src='https://mdbootstrap.com/img/new/standard/city/053.webp' className='img-fluid' />
<div className='mask' style={{ backgroundColor: doubleTapColor }}>
<div className='d-flex justify-content-center align-items-center h-100'>
<p className='text-white mb-0'>Change background color with 2 taps</p>
</div>
</div>
</div>
<div className='my-3'>
<MDBTouch taps={2} duration={2000} tag={MDBBtn} className='my-3' type='tap' onTap={handleDoubleTap}>
{' '}
Tap button
</MDBTouch>
</div>
</div>
);
}
Pan
The pan event is useful for dragging elements - every time the user moves a finger on the element to which the directive is attached to, the given method is being called with an argument consisting of two keys: x and y (the values correspond to the horizontal and vertical translation).
import React, { useState } from 'react';
import { MDBTouch } from 'mdb-react-ui-kit';
export default function App() {
const [moveElement, setMoveElement] = useState({ x: 0, y: 0 });
const [prevTouch, setPrevTouch] = useState<{ pageX: number | null; pageY: number | null }>({
pageX: null,
pageY: null,
});
const handlePan = (e: any) => {
const touch = e.touches[0];
if (prevTouch.pageX && prevTouch.pageY) {
e.movementX = touch.pageX - prevTouch.pageX;
e.movementY = touch.pageY - prevTouch.pageY;
setMoveElement({ x: moveElement.x + e.movementX, y: moveElement.y + e.movementY });
}
setPrevTouch(touch);
};
return (
<MDBTouch type='pan' allDirections onPan={(e: any) => handlePan(e)} tag='div'>
<div className='bg-image' id='div-pan'>
<img
src='https://mdbootstrap.com/img/new/standard/city/053.webp'
className='img-fluid'
id='img-pan'
style={{ transform: `translate(${moveElement.x}px, ${moveElement.y}px)` }}
/>
</div>
</MDBTouch>
);
}
Pan Left
Pan with only left direction
import React, { useState } from 'react';
import { MDBTouch } from 'mdb-react-ui-kit';
export default function App() {
const [moveLeft, setMoveLeft] = useState({ x: 0 });
const [prevLeft, setPrevLeft] = useState<{ pageX: number | null }>({ pageX: null });
const handlePanLeft = (e: any) => {
const touch = e.touches[0];
if (prevLeft.pageX) {
e.movementX = touch.pageX - prevLeft.pageX;
setMoveLeft({ x: moveLeft.x + e.movementX });
}
setPrevLeft(touch);
};
return (
<MDBTouch type='pan' onPanLeft={(e: any) => handlePanLeft(e)} tag='div'>
<div className='bg-image'>
<img
src='https://mdbootstrap.com/img/new/standard/city/053.webp'
className='img-fluid'
id='img-pan'
style={{ transform: `translate(${moveLeft.x}px, 0px)` }}
/>
</div>
</MDBTouch>
);
}
Pan Right
Pan with only right direction
import React, { useState } from 'react';
import { MDBTouch } from 'mdb-react-ui-kit';
export default function App() {
const [moveRight, setMoveRight] = useState({ x: 0 });
const [prevRight, setPrevRight] = useState<{ pageX: number | null }>({ pageX: null });
const handlePanRight = (e: any) => {
const touch = e.touches[0];
if (prevRight.pageX) {
e.movementX = touch.pageX - prevRight.pageX;
setMoveRight({ x: moveRight.x + e.movementX });
}
setPrevRight(touch);
};
return (
<MDBTouch type='pan' onPanRight={(e: any) => handlePanRight(e)} tag='div'>
<div className='bg-image'>
<img
src='https://mdbootstrap.com/img/new/standard/city/053.webp'
className='img-fluid'
id='img-pan'
style={{ transform: `translate(${moveRight.x}px, 0px)` }}
/>
</div>
</MDBTouch>
);
}
Pan Up/Down
Pan with only up/down direction
import React, { useState } from 'react';
import { MDBTouch } from 'mdb-react-ui-kit';
export default function App() {
const [moveY, setMoveY] = useState({ y: 0 });
const [prevY, setPrevY] = useState<{ pageY: number | null }>({ pageY: null });
const handlePanY = (e: any) => {
const touch = e.touches[0];
if (prevY.pageY) {
e.movementY = touch.pageY - prevY.pageY;
setMoveY({ y: moveY.y + e.movementY });
}
setPrevY(touch);
};
return (
<MDBTouch type='pan' onPanUp={(e: any) => handlePanY(e)} onPanDown={(e: any) => handlePanY(e)} tag='div'>
<div className='bg-image'>
<img
src='https://mdbootstrap.com/img/new/standard/city/053.webp'
className='img-fluid'
id='img-pan'
style={{ transform: `translate(0px, ${moveY.y}px)` }}
/>
</div>
</MDBTouch>
);
}
Pinch
The pinch event calls the given method with an object containing two keys - ratio and origin. The first one it the ratio of the distance between user's fingers on touchend to the same distance on touchstart - it's particularly useful for scaling images. The second one, similarly as in doubleTap event, is a pair of coordinates indicating the middle point between the user's fingers.
import React, { useState } from 'react';
import { MDBTouch } from 'mdb-react-ui-kit';
export default function App() {
const [origin1, setOrigin1] = useState<{ x: number | null; y: number | null }>({ x: null, y: null });
const handlePinch = (e: any, data: any) => {
const { origin, ratio } = data;
const { target } = e;
let scale = 1;
const { x, y } = target.getBoundingClientRect();
setOrigin1({ x: origin.x - x, y: origin.y - y });
scale * ratio > 1 ? (scale *= ratio) : (scale = 1);
target.style.transform = `scale(${scale})`;
target.style.transformOrigin = origin1 ? `${origin1.x}px ${origin1.y}px 0` : '50% 50% 0';
};
return (
<MDBTouch type='pinch' pointers={2} onPinch={(e: any, data: any) => handlePinch(e, data)} tag='div'>
<img src='https://mdbootstrap.com/img/new/standard/city/053.webp' className='img-fluid' id='img-pinch' />
</MDBTouch>
);
}
Swipe Left/Right
The swipe event comes with several modifiers (left, right, up, down) - each of them will ensure that event will fire only on swipe in that particular direction. If the directive is used without any modifier, the callback method will be called each time the swiping occurs, and the string indicating the direction will be passed as an argument.
This example shows example with left and right
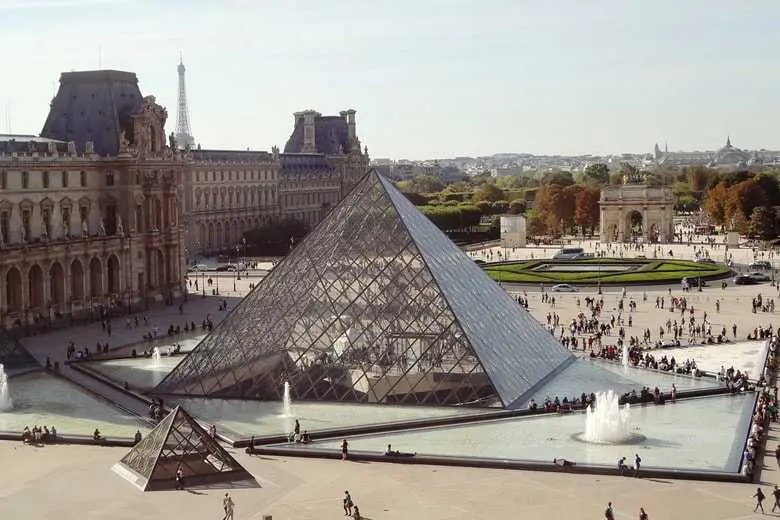
Swipe Left-Right to change a color
import React, { useState } from 'react';
import { MDBTouch } from 'mdb-react-ui-kit';
export default function App() {
const [bgXColor, setBgXColor] = useState('rgba(0, 0, 0, 0.6)');
const colorGen = () => {
const randomNum = () => {
return Math.floor(Math.random() * 255) + 1;
};
return `rgba(${randomNum()},${randomNum()},${randomNum()},.4)`;
};
const handleSwipe = () => {
setBgXColor(colorGen());
};
return (
<MDBTouch
type='swipe'
onSwipeLeft={handleSwipe}
onSwipeRight={handleSwipe}
threshold={200}
tag='div'
>
<div className='bg-image'>
<img src='https://mdbootstrap.com/img/new/standard/city/053.webp' className='img-fluid' />
<div className='mask' style={{ backgroundColor: bgXColor }}>
<div className='d-flex justify-content-center align-items-center h-100'>
<p className='text-white mb-0'>Swipe Left-Right to change a color</p>
</div>
</div>
</div>
</MDBTouch>
);
}
Swipe Up/Down
This example shows example with up and down
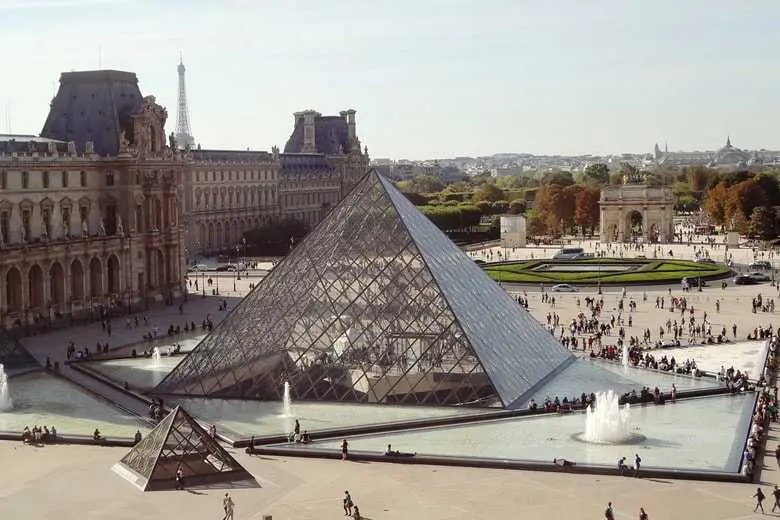
Swipe Up-Down to change a color
import React, { useState } from 'react';
import { MDBTouch } from 'mdb-react-ui-kit';
export default function App() {
const [bgYColor, setBgYColor] = useState('rgba(0, 0, 0, 0.6)');
const colorGen = () => {
const randomNum = () => {
return Math.floor(Math.random() * 255) + 1;
};
return `rgba(${randomNum()},${randomNum()},${randomNum()},.4)`;
};
const handleSwipe = () => {
setBgYColor(colorGen());
};
return (
<MDBTouch
type='swipe'
onSwipeUp={handleSwipe}
onSwipeDown={handleSwipe}
threshold={200}
tag='div'
>
<div className='bg-image'>
<img src='https://mdbootstrap.com/img/new/standard/city/053.webp' className='img-fluid' />
<div className='mask' style={{ backgroundColor: bgYColor }}>
<div className='d-flex justify-content-center align-items-center h-100'>
<p className='text-white mb-0'>Swipe Up-Down to change a color</p>
</div>
</div>
</div>
</MDBTouch>
);
}
Rotate
This example shows example with rotate
import React, { useState } from 'react';
import { MDBTouch } from 'mdb-react-ui-kit';
export default function App() {
const [currentAngle, setCurrentAngle] = useState(0);
const handleRotate = (e: any, data: any) => {
setCurrentAngle(currentAngle + data.distance);
e.target.style.transform = `rotate(${currentAngle}deg)`;
};
return (
<MDBTouch
pointers={2}
threshold={30}
type='rotate'
onRotate={(e: any, data: any) => handleRotate(e, data)}
className='bg-image'
>
<img src='https://mdbootstrap.com/img/new/standard/city/053.webp' className='img-fluid' id='img-rotate' />
</MDBTouch>
);
}
Touch - API
Import
import { MDBTouch } from 'mdb-react-ui-kit';
Properties
MDBTouch
Name | Type | Default | Description |
---|---|---|---|
tag
|
String | 'div' |
Defines tag of the MDBTouch element |
className
|
String | '' |
Add custom class to MDBTouch |
touchRef
|
Reference | '' |
A reference for the MDBTouch element |
type
|
'swipe' | 'tap' | 'press' | 'pan' | 'pinch' | 'rotate' | '' |
A type of touch event |
Tap
Name | Type | Default | Description |
---|---|---|---|
interval
|
number | 500 |
Set interval to tap |
time
|
number | 250 |
Set delays time to tap event |
taps
|
number | 1 |
Set default value of number for taps |
pointers
|
number | 1 |
Set default value of number for pointers |
onTap
|
Function |
|
A callback for tap event |
Press
Name | Type | Default | Description |
---|---|---|---|
time
|
number | 250 |
Set time delays to take tap |
pointers
|
number | 1 |
Set default value of number for pointers |
onPress
|
Function |
|
A callback for press event |
Swipe
Name | Type | Default | Description |
---|---|---|---|
threshold
|
number | 10 |
Set distance bettwen when event fires |
direction
|
string | all |
Set direction to swipe. Available options: all, right, left, top, up. |
allDirections
|
boolean | true |
Enables swiping in all directions |
onSwipe
|
Function |
|
A callback for swipe event (in all directions) |
onSwipeLeft
|
Function |
|
A callback for swipe event (in the left direction) |
onSwipeRight
|
Function |
|
A callback for swipe event (in the right direction) |
onSwipeUp
|
Function |
|
A callback for swipe event (in the up direction) |
onSwipeDown
|
Function |
|
A callback for swipe event (in the down direction) |
Rotate
Name | Type | Default | Description |
---|---|---|---|
angle
|
number | 0 |
Set started angle to rotate. |
pointers
|
number | 2 |
Set default value of number for pointers |
onRotate
|
Function |
|
A callback for rotate event |
Pan
Name | Type | Default | Description |
---|---|---|---|
threshold
|
number | 20 |
Set distance bettwen when event fires |
direction
|
string | all |
Set direction to pan. Available options: all, right, left, top, up. |
pointers
|
number | 1 |
Set default value of number for pointers |
allDirections
|
boolean | true |
Enables pan event in all directions |
onPan
|
Function |
|
A callback for pan event (in all directions) |
onPanLeft
|
Function |
|
A callback for pan event (in the left direction) |
onPanRight
|
Function |
|
A callback for pan event (in the right direction) |
onPanUp
|
Function |
|
A callback for pan event (in the up direction) |
onPanDown
|
Function |
|
A callback for pan event (in the down direction) |
Pinch
Name | Type | Default | Description |
---|---|---|---|
pointers
|
number | 2 |
Option for tap event, set duration to how long it
takes to take a tap
|
threshold
|
number | 10 |
Set distance bettwen when event fires |
onPinch
|
Function |
|
A callback for pinch event |