Sticky
React Bootstrap 5 Sticky
Sticky is a component which allows elements to be locked in a particular area of the page. It is often used in navigation menus.
Note: Read the API tab to find all available options and advanced customization
Basic example
To start use sticky just create MDBSticky
component
import React from 'react';
import { MDBSticky } from 'mdb-react-ui-kit';
export default function App() {
return (
<MDBSticky
boundary
tag='a'
className='btn btn-outline-primary bg-white'
href='https://mdbootstrap.com/docs/jquery/getting-started/download/'
>
Download MDB
</MDBSticky>
);
}
Sticky bottom
You can pin element to bottom by adding
stickyPosition="bottom"
import React from 'react';
import { MDBSticky } from 'mdb-react-ui-kit';
export default function App() {
return (
<MDBSticky
boundary
position='bottom'
direction='both'
tag='img'
src='https://mdbcdn.b-cdn.net/wp-content/uploads/2018/06/logo-mdb-jquery-small.webp'
alt='MDB Logo'
/>
);
}
Boundary
Set boundary
so that sticky only works inside the parent
element. Remember to set the correct parent height.
import React from 'react';
import { MDBSticky } from 'mdb-react-ui-kit';
export default function App() {
return (
<MDBSticky
boundary
tag='img'
src='https://mdbcdn.b-cdn.net/wp-content/uploads/2018/06/logo-mdb-jquery-small.webp'
alt='MDB Logo'
/>
);
}
Outer element as a boundary
You can specify an element selector to be the sticky boundary.
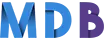
import React from 'react';
import { MDBSticky } from 'mdb-react-ui-kit';
export default function App() {
return (
<>
<div>
<MDBSticky
boundary='#sticky-stop'
direction='both'
tag='img'
src='https://mdbcdn.b-cdn.net/wp-content/uploads/2018/06/logo-mdb-jquery-small.webp'
alt='MDB Logo'
/>
</div>
<div id='sticky-stop' className='mt-auto' style={{ height: '5rem' }}>
Stop here
</div>
</>
);
}
Direction
Default direction of sticky component is down
. You can change it by setting
direction="top"
or
direction="both"
import React from 'react';
import { MDBSticky } from 'mdb-react-ui-kit';
export default function App() {
return (
<>
<MDBSticky boundary tag='a' className='btn btn btn-primary' direction='up'>
Up
</MDBSticky>
<MDBSticky boundary tag='a' className='btn btn btn-primary' direction='down'>
Down
</MDBSticky>
<MDBSticky boundary tag='a' className='btn btn btn-primary' direction='both'>
Both
</MDBSticky>
</>
);
}
Animation
You can add an animation that will run when the sticky starts and when the sticky element is
hidden. just specify the css class of the animation using the
stickyAnimationSticky
and
stickyAnimationUnsticky
properties.
Remember that not every animation will be appropriate. We suggest using the animations used in the example below.
import React from 'react';
import { MDBSticky } from 'mdb-react-ui-kit';
export default function App() {
return (
<MDBSticky
id='MDB-logo'
tag='img'
src='https://mdbcdn.b-cdn.net/wp-content/uploads/2018/06/logo-mdb-jquery-small.webp'
alt='MDB Logo'
animationSticky='slide-in-down'
animationUnsticky='slide-up'
boundary
/>
);
}
Sticky - API
Import
import { MDBSticky } from 'mdb-react-ui-kit';
Properties
MDBSticky
Name | Type | Default | Description | Example |
---|---|---|---|---|
className
|
string | '' |
Add custom class to MDBSticky |
<MDBSticky className="class" />
|
animationSticky
|
'fade-in' | 'fade-in-down' | 'fade-in-left' | 'fade-in-right' | 'fade-in-up' | 'fade-out' | 'fade-out-down' | 'fade-out-left' | 'fade-out-right' | 'fade-out-up' | 'slide-in-down' | 'slide-in-left' | 'slide-in-right' | 'slide-in-up' | 'slide-out-down' | 'slide-out-left' | 'slide-out-right' | 'slide-out-up' | 'slide-down' | 'slide-left' | 'slide-right' | 'slide-up' | 'zoom-in' | 'zoom-out' | 'tada' | 'pulse' | 'drop-in' | 'drop-out' | 'fly-in' | 'fly-in-up' | 'fly-in-down' | 'fly-in-left' | 'fly-in-right' | 'fly-out' | 'fly-out-up' | 'fly-out-down' | 'fly-out-left' | 'fly-out-right' | 'browse-in' | 'browse-out' | 'browse-out-left' | 'browse-out-right' | 'jiggle' | 'flash' | 'shake' | 'glow' | '' |
Set sticky animation |
<MDBSticky stickyAnimationSticky="fade-in" />
|
animationUnsticky
|
'fade-in' | 'fade-in-down' | 'fade-in-left' | 'fade-in-right' | 'fade-in-up' | 'fade-out' | 'fade-out-down' | 'fade-out-left' | 'fade-out-right' | 'fade-out-up' | 'slide-in-down' | 'slide-in-left' | 'slide-in-right' | 'slide-in-up' | 'slide-out-down' | 'slide-out-left' | 'slide-out-right' | 'slide-out-up' | 'slide-down' | 'slide-left' | 'slide-right' | 'slide-up' | 'zoom-in' | 'zoom-out' | 'tada' | 'pulse' | 'drop-in' | 'drop-out' | 'fly-in' | 'fly-in-up' | 'fly-in-down' | 'fly-in-left' | 'fly-in-right' | 'fly-out' | 'fly-out-up' | 'fly-out-down' | 'fly-out-left' | 'fly-out-right' | 'browse-in' | 'browse-out' | 'browse-out-left' | 'browse-out-right' | 'jiggle' | 'flash' | 'shake' | 'glow' | '' |
Set unsticky animation |
<MDBSticky stickyAnimationUnsticky="fade-out" />
|
boundary
|
boolean | false |
set to true to stop sticky on the end of the parent |
<MDBSticky stickyBoundary />
|
delay
|
number | 0 |
Set the number of pixels beyond which the item will be pinned |
<MDBSticky stickyDelay={100} />
|
direction
|
'up' | 'down' | 'both' | 'down' |
Set the scrolling direction for which the element is to be stikcky |
<MDBSticky stickyDirection='up' />
|
offset
|
Number | 0 |
Set the distance from the top edge of the element for which the element is sticky |
<MDBSticky stickyOffset={100} />
|
position
|
'top' | 'bottom' | 'top' |
Set the edge of the screen the item should stick to |
<MDBSticky stickyPosition="down" />
|