Images
React Bootstrap 5 Images component
Documentation and examples for opting images into responsive behavior (so they never become larger than their parent elements) and add lightweight styles to them—all via classes.
Responsive images
Images in MDB are made responsive with .img-fluid
. This applies
max-width: 100%;
and height: auto;
to the image so that it scales
with the parent element.
import React from 'react';
export default function App() {
return (
<img src='https://mdbootstrap.com/img/new/slides/041.webp' className='img-fluid shadow-4' alt='...' />
);
}
Thumbnails
In addition to our
border-radius utilities, you can use
.img-thumbnail
to give an image a rounded 1px border appearance.
import React from 'react';
export default function App() {
return (
<img
src='https://mdbootstrap.com/img/new/standard/city/041.webp'
className='img-thumbnail'
alt='...'
style={{ maxWidth: '24rem' }}
/>
);
}
Shadows
By using our
shadow classes you can add a shadow to
the image. In the example below, we add shadow-2-strong
class.
import React from 'react';
export default function App() {
return (
<img
src='https://mdbootstrap.com/img/new/standard/city/042.webp'
className='img-fluid shadow-2-strong'
alt=''
style={{ maxWidth: '24rem' }}
/>
);
}
By adding .hover-shadow
class to the element you can apply a shadow hover effect.
import React from 'react';
export default function App() {
return (
<img
src='https://mdbootstrap.com/img/new/standard/city/043.webp'
className='img-fluid hover-shadow'
alt=''
style={{ maxWidth: '24rem' }}
/>
);
}
Ripple
You can change the image into a clickable element and apply a
ripple effect to it by simply adding
.ripple
class.
import React from 'react';
import { MDBRipple } from 'mdb-react-ui-kit';
export default function App() {
return (
<MDBRipple rippleTag='a'>
<img
src='https://mdbootstrap.com/img/new/standard/city/044.webp'
className='img-fluid rounded'
alt='example'
style={{ maxWidth: '24rem' }}
/>
</MDBRipple>
);
}
Masks
You can cover the image with mask to achieve the desired contrast and for example, place text on it.
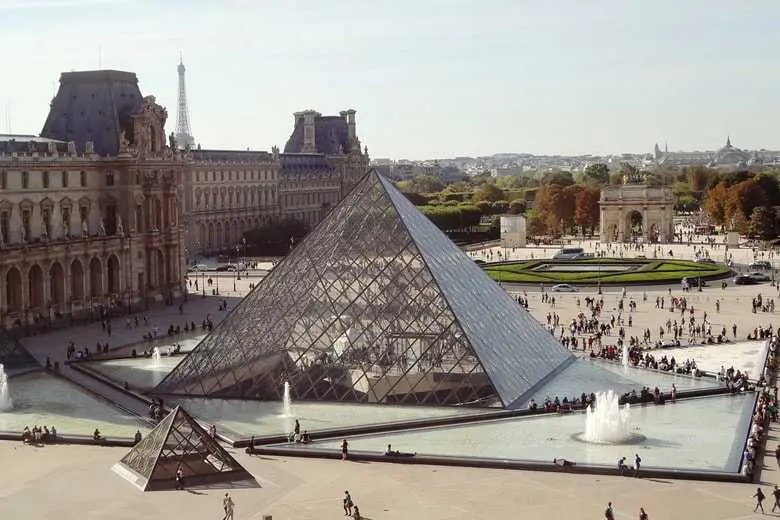
Can you see me?
import React from 'react';
export default function App() {
return (
<div className='bg-image' style={{ maxWidth: '24rem' }}>
<img src='https://mdbootstrap.com/img/new/standard/city/053.webp' className='img-fluid' alt='Sample' />
<div className='mask' style={{ backgroundColor: 'rgba(0, 0, 0, 0.6)' }}>
<div className='d-flex justify-content-center align-items-center h-100'>
<p className='text-white mb-0'>Can you see me?</p>
</div>
</div>
</div>
);
}
Hover effects
By using .hover-overlay
class you can apply gentle and decorative
hover effects to the image.
import React from 'react';
export default function App() {
return (
<div className='bg-image hover-overlay' style={{ maxWidth: '24rem' }}>
<img src='https://mdbootstrap.com/img/new/fluid/city/055.webp' className='img-fluid' />
<a href='#!'>
<div className='mask overlay' style={{ backgroundColor: 'rgba(57, 192, 237, 0.2)' }}></div>
</a>
</div>
);
}
Shapes
By using border utilities you can change the shape of the image.
.rounded
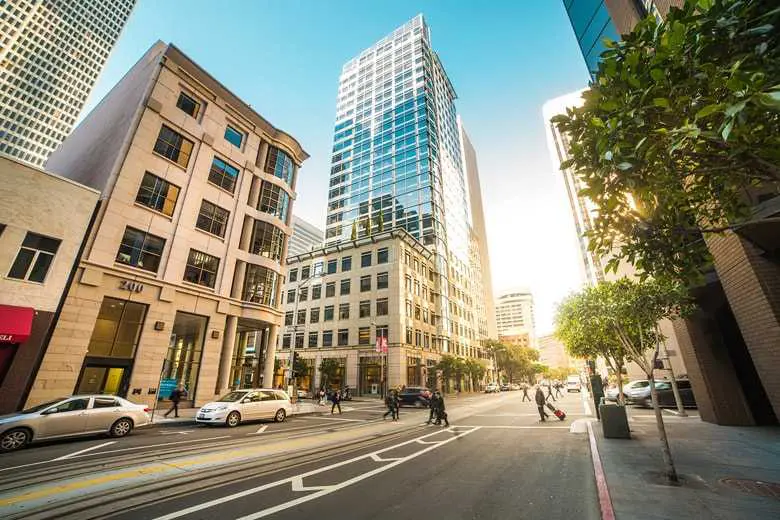
.rounded-circle
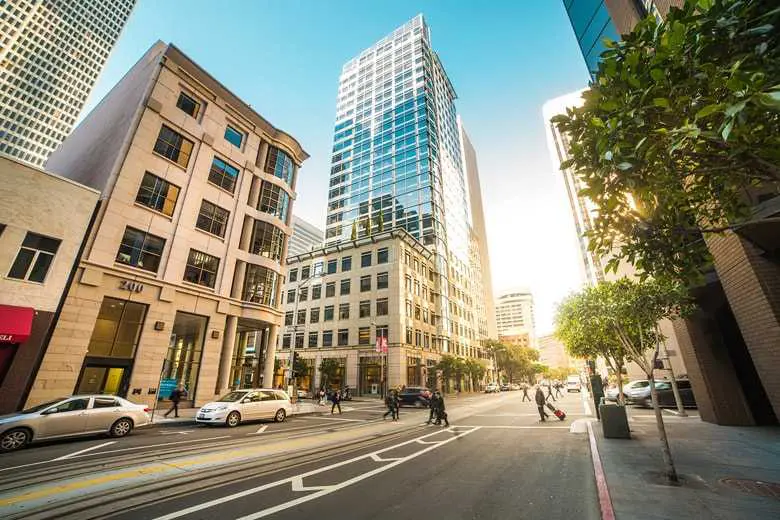
.rounded-pill
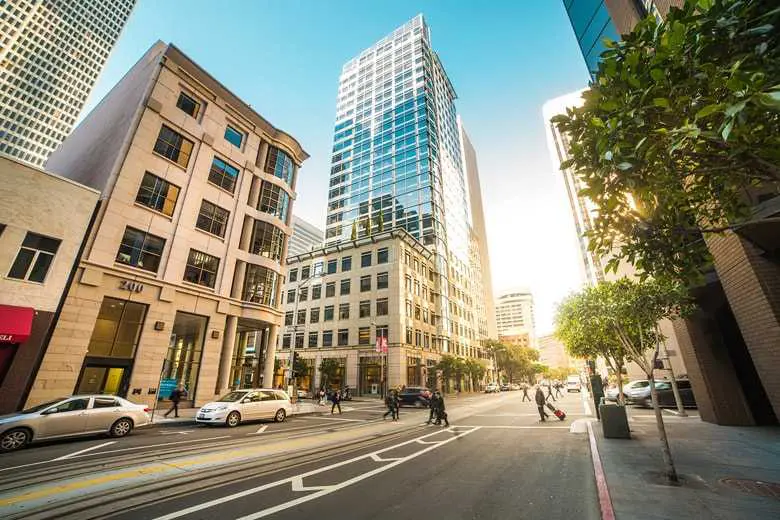
import React from 'react';
import { MDBRow, MDBCol } from 'mdb-react-ui-kit';
export default function App() {
return (
<MDBRow>
<MDBCol lg='4' md='12' className='mb-4'>
<img src='https://mdbootstrap.com/img/new/standard/city/047.webp' className='img-fluid rounded' alt='' />
</MDBCol>
<MDBCol lg='4' md='6' className='mb-4'>
<img
src='https://mdbootstrap.com/img/new/standard/city/047.webp'
className='img-fluid rounded-circle'
alt=''
/>
</MDBCol>
<MDBCol lg='4' md='6' className='mb-4'>
<img
src='https://mdbootstrap.com/img/new/standard/city/047.webp'
className='img-fluid rounded-pill'
alt=''
/>
</MDBCol>
</MDBRow>
);
}