Cards
React Bootstrap 5 Cards component
A card is a flexible and extensible content container. It includes options for headers and footers, a wide variety of content, contextual background colors, and powerful display options.
Basic examples
Note: Below are examples of basic cards with mixed content and a
fixed width. Cards have no fixed width to start, so they’ll naturally fill
the full width of its parent element.
To control the width of the card place it in the grid, use the sizing utilities, or set the
width inline. You can learn more in the
sizing section.
Simple
Card title
Some quick example text to build on the card title and make up the bulk of the card's content.
import React from 'react';
import { MDBCard, MDBCardBody, MDBCardTitle, MDBCardText, MDBBtn } from 'mdb-react-ui-kit';
export default function App() {
return (
<MDBCard style={{ maxWidth: '22rem' }}>
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
Some quick example text to build on the card title and make up the bulk of the card's content.
</MDBCardText>
<MDBBtn>Button</MDBBtn>
</MDBCardBody>
</MDBCard>
);
}
Image
import React from 'react';
import { MDBCard, MDBCardBody, MDBCardTitle, MDBCardText, MDBCardImage, MDBBtn } from 'mdb-react-ui-kit';
export default function App() {
return (
<MDBCard style={{ maxWidth: '22rem' }}>
<MDBCardImage src='https://mdbootstrap.com/img/new/standard/nature/184.webp' position='top' alt='...' />
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
Some quick example text to build on the card title and make up the bulk of the card's content.
</MDBCardText>
<MDBBtn href='#'>Button</MDBBtn>
</MDBCardBody>
</MDBCard>
);
}
Image with ripple
To add a ripple effect and subtle hover effect you need to modify the HTML markup of the card image.
import React from 'react';
import { MDBCard, MDBCardBody, MDBCardTitle, MDBCardText, MDBCardImage, MDBBtn, MDBRipple } from 'mdb-react-ui-kit';
export default function App() {
return (
<MDBCard style={{ maxWidth: '22rem' }}>
<MDBRipple rippleColor='light' rippleTag='div' className='bg-image hover-overlay'>
<MDBCardImage src='https://mdbootstrap.com/img/new/standard/nature/111.webp' fluid alt='...' />
<a>
<div className='mask' style={{ backgroundColor: 'rgba(251, 251, 251, 0.15)' }}></div>
</a>
</MDBRipple>
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
Some quick example text to build on the card title and make up the bulk of the card's content.
</MDBCardText>
<MDBBtn href='#'>Button</MDBBtn>
</MDBCardBody>
</MDBCard>
);
}
Content types
Cards support a wide variety of content, including images, text, list groups, links, and more. Below are examples of what’s supported.
Body
The building block of a card is the <MDBCardBody>
. Use it whenever you need a
padded section within a card.
import React from 'react';
import { MDBCard, MDBCardBody } from 'mdb-react-ui-kit';
export default function App() {
return (
<MDBCard>
<MDBCardBody>This is some text within a card body.</MDBCardBody>
</MDBCard>
);
}
Titles, text, and links
Card titles are used by using <MDBCardTitle>
with a tag="h*"
property. In
the same way, links are added and placed next to each other by using <MDBCardLink> component.
Subtitles are used by using <MDBCardSubTitle>
with a tag="h*"
property.
If the <MDBCardTitle>
and the <MDBCardSubTitle>
items are placed in a
<MDBCardBody>
item, the card title and subtitle are aligned nicely.
Card title
Card subtitle
Some quick example text to build on the card title and make up the bulk of the card's content.
Card link Another link
import React from 'react';
import { MDBCard, MDBCardBody, MDBCardTitle, MDBCardSubTitle, MDBCardText, MDBCardLink } from 'mdb-react-ui-kit';
export default function App() {
return (
<MDBCard style={{ width: '18rem' }}>
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardSubTitle>Card subtitle</MDBCardSubTitle>
<MDBCardText>
Some quick example text to build on the card title and make up the bulk of the card's content.
</MDBCardText>
<MDBCardLink href='#'>Card link</MDBCardLink>
<MDBCardLink href='#'>Another link</MDBCardLink>
</MDBCardBody>
</MDBCard>
);
}
Images
position="top"
places an image to the top of the card. With
<MDBCardText>
, text can be added to the card. Text within
<MDBCardText>
can also be styled with the standard HTML tags.
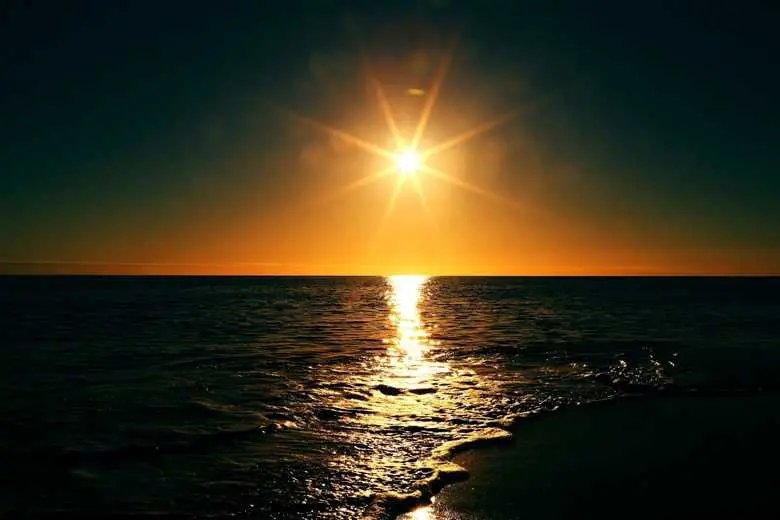
Some quick example text to build on the card title and make up the bulk of the card's content.
import React from 'react';
import { MDBCard, MDBCardBody, MDBCardText, MDBCardImage } from 'mdb-react-ui-kit';
export default function App() {
return (
<MDBCard style={{ width: '18rem' }}>
<MDBCardImage src='https://mdbootstrap.com/img/new/standard/nature/182.webp' alt='...' position='top' />
<MDBCardBody>
<MDBCardText>
Some quick example text to build on the card title and make up the bulk of the card's content.
</MDBCardText>
</MDBCardBody>
</MDBCard>
);
}
List groups
Create lists of content in a card with a flush list group.
- Cras justo odio
- Dapibus ac facilisis in
- Vestibulum at eros
import React from 'react';
import { MDBCard, MDBListGroup, MDBListGroupItem } from 'mdb-react-ui-kit';
export default function App() {
return (
<MDBCard style={{ width: '18rem' }}>
<MDBListGroup flush>
<MDBListGroupItem>Cras justo odio</MDBListGroupItem>
<MDBListGroupItem>Dapibus ac facilisis in</MDBListGroupItem>
<MDBListGroupItem>Vestibulum at eros</MDBListGroupItem>
</MDBListGroup>
</MDBCard>
);
}
- Cras justo odio
- Dapibus ac facilisis in
- Vestibulum at eros
import React from 'react';
import { MDBCard, MDBCardHeader, MDBListGroup, MDBListGroupItem } from 'mdb-react-ui-kit';
export default function App() {
return (
<MDBCard style={{ width: '18rem' }}>
<MDBCardHeader>Featured</MDBCardHeader>
<MDBListGroup flush>
<MDBListGroupItem>Cras justo odio</MDBListGroupItem>
<MDBListGroupItem>Dapibus ac facilisis in</MDBListGroupItem>
<MDBListGroupItem>Vestibulum at eros</MDBListGroupItem>
</MDBListGroup>
</MDBCard>
);
}
- Cras justo odio
- Dapibus ac facilisis in
- Vestibulum at eros
import React from 'react';
import { MDBCard, MDBCardFooter } from 'mdb-react-ui-kit';
export default function App() {
return (
<MDBCard style={{ width: '18rem' }}>
<MDBListGroup flush>
<MDBListGroupItem>Cras justo odio</MDBListGroupItem>
<MDBListGroupItem>Dapibus ac facilisis in</MDBListGroupItem>
<MDBListGroupItem>Vestibulum at eros</MDBListGroupItem>
</MDBListGroup>
<MDBCardFooter>Card footer</MDBCardFooter>
</MDBCard>
);
}
Kitchen sink
Mix and match multiple content types to create the card you need, or throw everything in there. Shown below are image styles, blocks, text styles, and a list group—all wrapped in a fixed-width card.
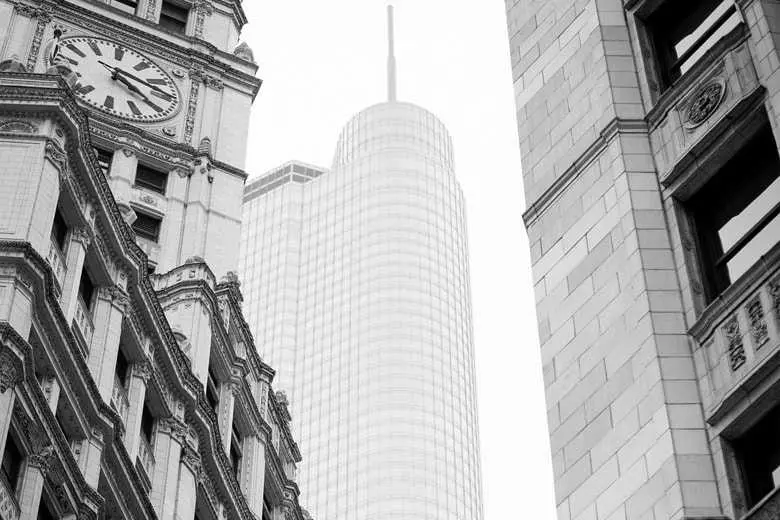
Card title
Some quick example text to build on the card title and make up the bulk of the card's content.
- Cras justo odio
- Dapibus ac facilisis in
- Vestibulum at eros
import React from 'react';
import { MDBCard, MDBCardImage, MDBCardBody, MDBCardTitle, MDBCardText, MDBCardLink, MDBListGroup, MDBListGroupItem } from 'mdb-react-ui-kit';
export default function App() {
return (
<MDBCard style={{ width: '18rem' }}>
<MDBCardImage position='top' alt='...' src='https://mdbootstrap.com/img/new/standard/city/062.webp' />
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
Some quick example text to build on the card title and make up the bulk of the card's content.
</MDBCardText>
</MDBCardBody>
<MDBListGroup flush>
<MDBListGroupItem>Cras justo odio</MDBListGroupItem>
<MDBListGroupItem>Dapibus ac facilisis in</MDBListGroupItem>
<MDBListGroupItem>Vestibulum at eros</MDBListGroupItem>
</MDBListGroup>
<MDBCardBody>
<MDBCardLink href='#'>Card link</MDBCardLink>
<MDBCardLink href='#'>Card link</MDBCardLink>
</MDBCardBody>
</MDBCard>
);
}
Sizing
Cards assume no specific width
to start, so they’ll be 100% wide unless
otherwise stated. You can change this as needed with custom CSS, grid classes, grid Sass
mixins, or utilities.
Using grid markup
Using the grid, wrap cards in columns and rows as needed.
Special title treatment
With supporting text below as a natural lead-in to additional content.
Go somewhereSpecial title treatment
With supporting text below as a natural lead-in to additional content.
Go somewhere
import React from 'react';
import { MDBCard, MDBCardBody, MDBCardTitle, MDBCardText, MDBRow, MDBCol, MDBBtn } from 'mdb-react-ui-kit';
export default function App() {
return (
<MDBRow>
<MDBCol sm='6'>
<MDBCard>
<MDBCardBody>
<MDBCardTitle>Special title treatment</MDBCardTitle>
<MDBCardText>
With supporting text below as a natural lead-in to additional content.
</MDBCardText>
<MDBBtn href='#'>Go somewhere</MDBBtn>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol sm='6'>
<MDBCard>
<MDBCardBody>
<MDBCardTitle>Special title treatment</MDBCardTitle>
<MDBCardText>
With supporting text below as a natural lead-in to additional content.
</MDBCardText>
<MDBBtn href='#'>Go somewhere</MDBBtn>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
);
}
Using utilities
Use our handful of available sizing utilities to quickly set a card’s width.
import React from 'react';
import { MDBCard, MDBCardBody, MDBCardTitle, MDBCardText, MDBBtn } from 'mdb-react-ui-kit';
export default function App() {
return (
<>
<MDBCard className='w-75'>
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>With supporting text below as a natural lead-in to additional content.</MDBCardText>
<MDBBtn href='#'>Button</MDBBtn>
</MDBCardBody>
</MDBCard>
<br />
<MDBCard className='w-50'>
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>With supporting text below as a natural lead-in to additional content.</MDBCardText>
<MDBBtn href='#'>Button</MDBBtn>
</MDBCardBody>
</MDBCard>
</>
);
}
Using custom CSS
Use custom CSS in your stylesheets or as inline styles to set a width.
Special title treatment
With supporting text below as a natural lead-in to additional content.
Go somewhere
import React from 'react';
import { MDBCard, MDBCardBody, MDBCardTitle, MDBCardText, MDBBtn } from 'mdb-react-ui-kit';
export default function App() {
return (
<MDBCard style={{ width: '18rem' }}>
<MDBCardBody>
<MDBCardTitle>Special title treatment</MDBCardTitle>
<MDBCardText>With supporting text below as a natural lead-in to additional content.</MDBCardText>
<MDBBtn href='#'>Go somewhere</MDBBtn>
</MDBCardBody>
</MDBCard>
);
}
Text alignment
You can quickly change the text alignment of any card—in its entirety or specific parts—with our text align classes.
Special title treatment
With supporting text below as a natural lead-in to additional content.
Go somewhereSpecial title treatment
With supporting text below as a natural lead-in to additional content.
Go somewhereSpecial title treatment
With supporting text below as a natural lead-in to additional content.
Go somewhere
import React from 'react';
import { MDBCard, MDBCardBody, MDBCardTitle, MDBCardText, MDBBtn } from 'mdb-react-ui-kit';
export default function App() {
return (
<>
<MDBCard style={{ width: '18rem' }}>
<MDBCardBody>
<MDBCardTitle>Special title treatment</MDBCardTitle>
<MDBCardText>With supporting text below as a natural lead-in to additional content.</MDBCardText>
<MDBBtn href='#'>Go somewhere</MDBBtn>
</MDBCardBody>
</MDBCard>
<br />
<MDBCard style={{ width: '18rem' }} alignment='center'>
<MDBCardBody>
<MDBCardTitle>Special title treatment</MDBCardTitle>
<MDBCardText>With supporting text below as a natural lead-in to additional content.</MDBCardText>
<MDBBtn href='#'>Go somewhere</MDBBtn>
</MDBCardBody>
</MDBCard>
<br />
<MDBCard style={{ width: '18rem' }} alignment='end'>
<MDBCardBody>
<MDBCardTitle>Special title treatment</MDBCardTitle>
<MDBCardText>With supporting text below as a natural lead-in to additional content.</MDBCardText>
<MDBBtn href='#'>Go somewhere</MDBBtn>
</MDBCardBody>
</MDBCard>
</>
);
}
Images
Cards include a few options for working with images. Choose from appending “image caps” at either end of a card, overlaying images with card content, or simply embedding the image in a card.
Image caps
Similar to headers and footers, cards can include top and bottom “image caps”—images at the top or bottom of a card.
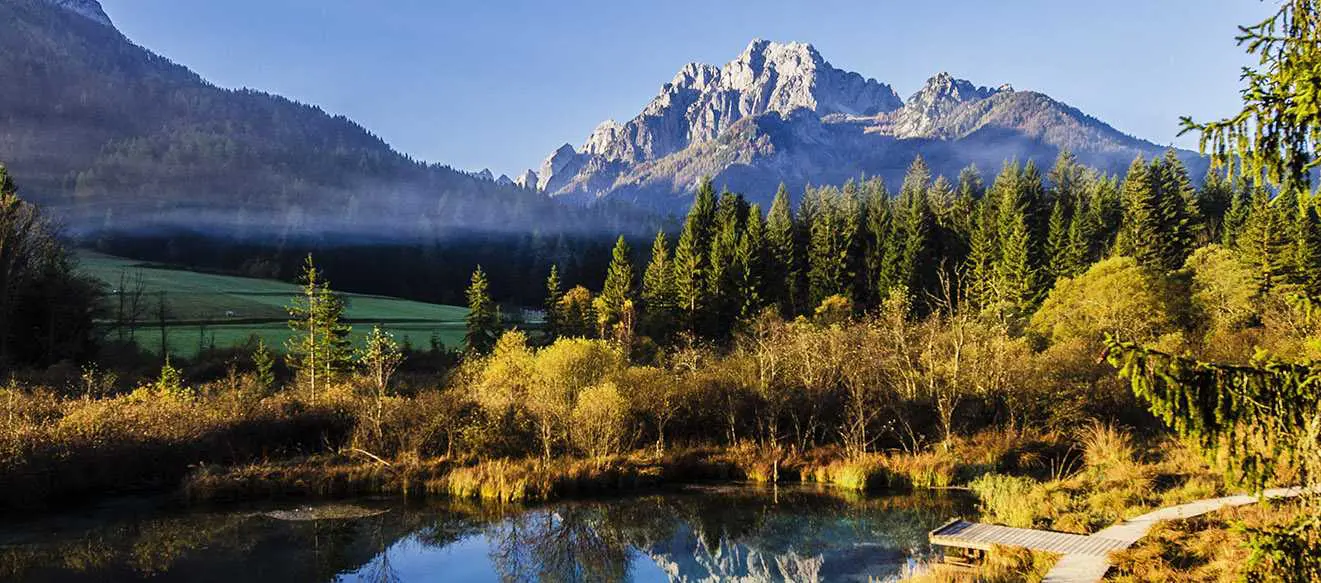
Card title
This is a wider card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.
Last updated 3 mins ago
Card title
This is a wider card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.
Last updated 3 mins ago
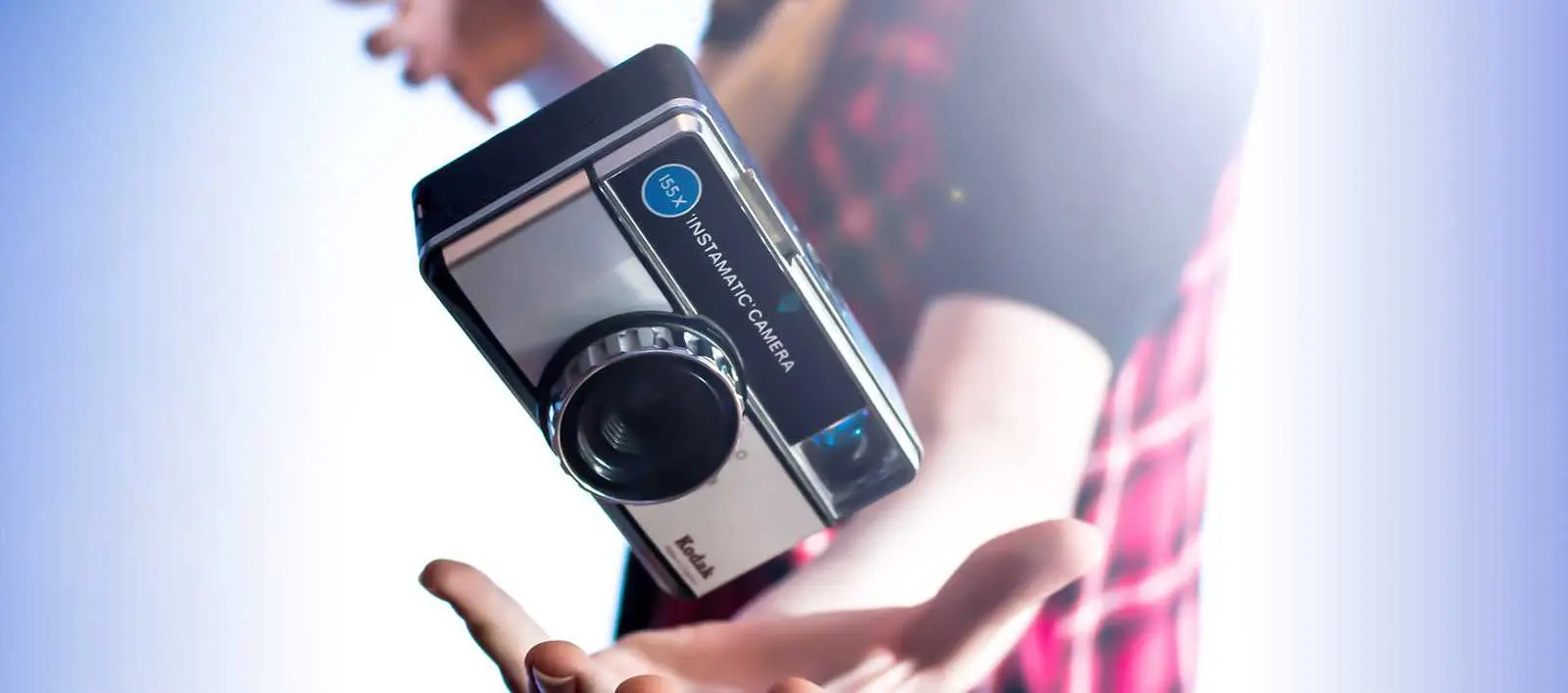
import React from 'react';
import { MDBCard, MDBCardBody, MDBCardTitle, MDBCardText, MDBCardImage } from 'mdb-react-ui-kit';
export default function App() {
return (
<>
<MDBCard className='mb-3'>
<MDBCardImage position='top' src='https://mdbootstrap.com/img/new/slides/041.webp' alt='...' />
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This is a wider card with supporting text below as a natural lead-in to additional content. This
content is a little bit longer.
</MDBCardText>
<MDBCardText>
<small className='text-muted'>Last updated 3 mins ago</small>
</MDBCardText>
</MDBCardBody>
</MDBCard>
<MDBCard className='mb-3'>
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This is a wider card with supporting text below as a natural lead-in to additional content. This
content is a little bit longer.
</MDBCardText>
<MDBCardText>
<small className='text-muted'>Last updated 3 mins ago</small>
</MDBCardText>
</MDBCardBody>
<MDBCardImage position='bottom' src='https://mdbootstrap.com/img/new/slides/042.webp' alt='...' />
</MDBCard>
</>
);
}
Image overlays
Turn an image into a card background and overlay your card’s text. Depending on the image, you may or may not need additional styles or utilities.
import React from 'react';
import { MDBCard, MDBCardTitle, MDBCardText, MDBCardOverlay, MDBCardImage } from 'mdb-react-ui-kit';
export default function App() {
return (
<MDBCard background='dark' className='text-white'>
<MDBCardImage overlay src='https://mdbootstrap.com/img/new/slides/017.webp' alt='...' />
<MDBCardOverlay>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This is a wider card with supporting text below as a natural lead-in to additional content. This
content is a little bit longer.
</MDBCardText>
<MDBCardText>Last updated 3 mins ago</MDBCardText>
</MDBCardOverlay>
</MDBCard>
);
}
Note: Content should not be larger than the height of the image. If content is larger than the image the content will be displayed outside the image.
Horizontal
Using a combination of grid and utility classes, cards can be made horizontal in a
mobile-friendly and responsive way. In the example below, we remove the grid gutters with
.g-0
and use .col-md-*
classNames to make the card horizontal at the
md
breakpoint. Further adjustments may be needed depending on your card content.
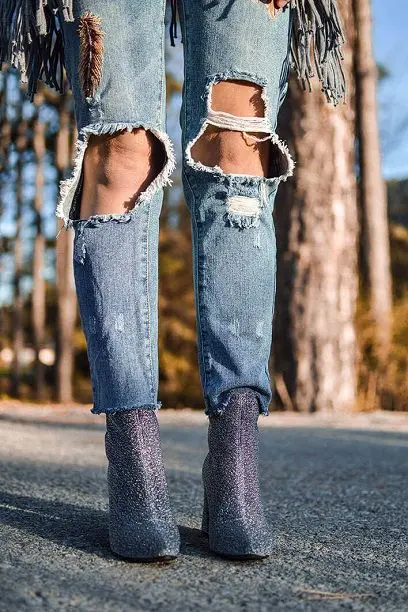
Card title
This is a wider card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.
Last updated 3 mins ago
import React from 'react';
import { MDBCard, MDBCardTitle, MDBCardText, MDBCardBody, MDBCardImage, MDBRow, MDBCol } from 'mdb-react-ui-kit';
export default function App() {
return (
<MDBCard style={{ maxWidth: '540px' }}>
<MDBRow className='g-0'>
<MDBCol md='4'>
<MDBCardImage src='https://mdbootstrap.com/wp-content/uploads/2020/06/vertical.webp' alt='...' fluid />
</MDBCol>
<MDBCol md='8'>
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This is a wider card with supporting text below as a natural lead-in to additional content. This
content is a little bit longer.
</MDBCardText>
<MDBCardText>
<small className='text-muted'>Last updated 3 mins ago</small>
</MDBCardText>
</MDBCardBody>
</MDBCol>
</MDBRow>
</MDBCard>
);
}
Card styles
Cards include various options for customizing their backgrounds, borders, and color.
Background and color
Use text and background utilities to change the appearance of a card.
Primary card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Secondary card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Success card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Danger card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Warning card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Info card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Light card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Dark card title
Some quick example text to build on the card title and make up the bulk of the card's content.
import React from 'react';
import { MDBCard, MDBCardTitle, MDBCardText, MDBCardBody, MDBCardHeader } from 'mdb-react-ui-kit';
export default function App() {
return (
<div>
<MDBCard background='primary' className='text-white mb-3' style={{ maxWidth: '18rem' }}>
<MDBCardHeader>Header</MDBCardHeader>
<MDBCardBody>
<MDBCardTitle>Primary card title</MDBCardTitle>
<MDBCardText>
Some quick example text to build on the card title and make up the bulk of the card's content.
</MDBCardText>
</MDBCardBody>
</MDBCard>
<MDBCard background='secondary' className='text-white mb-3' style={{ maxWidth: '18rem' }}>
<MDBCardHeader>Header</MDBCardHeader>
<MDBCardBody>
<MDBCardTitle>Secondary card title</MDBCardTitle>
<MDBCardText>
Some quick example text to build on the card title and make up the bulk of the card's content.
</MDBCardText>
</MDBCardBody>
</MDBCard>
<MDBCard background='success' className='text-white mb-3' style={{ maxWidth: '18rem' }}>
<MDBCardHeader>Header</MDBCardHeader>
<MDBCardBody>
<MDBCardTitle>Success card title</MDBCardTitle>
<MDBCardText>
Some quick example text to build on the card title and make up the bulk of the card's content.
</MDBCardText>
</MDBCardBody>
</MDBCard>
<MDBCard background='danger' className='text-white mb-3' style={{ maxWidth: '18rem' }}>
<MDBCardHeader>Header</MDBCardHeader>
<MDBCardBody>
<MDBCardTitle>Danger card title</MDBCardTitle>
<MDBCardText>
Some quick example text to build on the card title and make up the bulk of the card's content.
</MDBCardText>
</MDBCardBody>
</MDBCard>
<MDBCard background='warning' className='mb-3' style={{ maxWidth: '18rem' }}>
<MDBCardHeader>Header</MDBCardHeader>
<MDBCardBody>
<MDBCardTitle>Warning card title</MDBCardTitle>
<MDBCardText>
Some quick example text to build on the card title and make up the bulk of the card's content.
</MDBCardText>
</MDBCardBody>
</MDBCard>
<MDBCard background='info' className='text-body mb-3' style={{ maxWidth: '18rem' }}>
<MDBCardHeader>Header</MDBCardHeader>
<MDBCardBody>
<MDBCardTitle>Info card title</MDBCardTitle>
<MDBCardText>
Some quick example text to build on the card title and make up the bulk of the card's content.
</MDBCardText>
</MDBCardBody>
</MDBCard>
<MDBCard background='light' className='mb-3' style={{ maxWidth: '18rem' }}>
<MDBCardHeader>Header</MDBCardHeader>
<MDBCardBody>
<MDBCardTitle>Light card title</MDBCardTitle>
<MDBCardText>
Some quick example text to build on the card title and make up the bulk of the card's content.
</MDBCardText>
</MDBCardBody>
</MDBCard>
<MDBCard background='dark' className='text-white' style={{ maxWidth: '18rem' }}>
<MDBCardHeader>Header</MDBCardHeader>
<MDBCardBody>
<MDBCardTitle>Dark card title</MDBCardTitle>
<MDBCardText>
Some quick example text to build on the card title and make up the bulk of the card's content.
</MDBCardText>
</MDBCardBody>
</MDBCard>
</div>
);
}
Conveying meaning to assistive technologies:
Using color to add meaning only provides a visual indication, which will not be conveyed to
users of assistive technologies – such as screen readers. Ensure that information denoted by
the color is either obvious from the content itself (e.g. the visible text), or is included
through alternative means, such as additional text hidden with the
.visually-hidden
class.
Border
Use border utilities to change just the
border-color
of a card. Note that you can put
.text-{color}
classNames on the parent <MDBCard>
or a subset of the
card’s contents as shown below.
Primary card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Secondary card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Success card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Danger card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Warning card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Info card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Light card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Dark card title
Some quick example text to build on the card title and make up the bulk of the card's content.
import React from 'react';
import { MDBCard, MDBCardTitle, MDBCardText, MDBCardBody, MDBCardHeader } from 'mdb-react-ui-kit';
export default function App() {
return (
<div>
<MDBCard shadow='0' border='primary' background='white' className='mb-3' style={{ maxWidth: '18rem' }}>
<MDBCardHeader>Header</MDBCardHeader>
<MDBCardBody className='text-primary'>
<MDBCardTitle>Primary card title</MDBCardTitle>
<MDBCardText>
Some quick example text to build on the card title and make up the bulk of the card's content.
</MDBCardText>
</MDBCardBody>
</MDBCard>
<MDBCard
shadow='0'
border='secondary'
background='white'
className='mb-3'
style={{ maxWidth: '18rem' }}
>
<MDBCardHeader>Header</MDBCardHeader>
<MDBCardBody className='text-secondary'>
<MDBCardTitle>Secondary card title</MDBCardTitle>
<MDBCardText>
Some quick example text to build on the card title and make up the bulk of the card's content.
</MDBCardText>
</MDBCardBody>
</MDBCard>
<MDBCard shadow='0' border='success' background='white' className='mb-3' style={{ maxWidth: '18rem' }}>
<MDBCardHeader>Header</MDBCardHeader>
<MDBCardBody className='text-success'>
<MDBCardTitle>Success card title</MDBCardTitle>
<MDBCardText>
Some quick example text to build on the card title and make up the bulk of the card's content.
</MDBCardText>
</MDBCardBody>
</MDBCard>
<MDBCard shadow='0' border='danger' background='white' className='mb-3' style={{ maxWidth: '18rem' }}>
<MDBCardHeader>Header</MDBCardHeader>
<MDBCardBody className='text-danger'>
<MDBCardTitle>Danger card title</MDBCardTitle>
<MDBCardText>
Some quick example text to build on the card title and make up the bulk of the card's content.
</MDBCardText>
</MDBCardBody>
</MDBCard>
<MDBCard shadow='0' border='warning' background='white' className='mb-3' style={{ maxWidth: '18rem' }}>
<MDBCardHeader>Header</MDBCardHeader>
<MDBCardBody>
<MDBCardTitle>Warning card title</MDBCardTitle>
<MDBCardText>
Some quick example text to build on the card title and make up the bulk of the card's content.
</MDBCardText>
</MDBCardBody>
</MDBCard>
<MDBCard shadow='0' border='info' background='white' className='mb-3' style={{ maxWidth: '18rem' }}>
<MDBCardHeader>Header</MDBCardHeader>
<MDBCardBody>
<MDBCardTitle>Info card title</MDBCardTitle>
<MDBCardText>
Some quick example text to build on the card title and make up the bulk of the card's content.
</MDBCardText>
</MDBCardBody>
</MDBCard>
<MDBCard shadow='0' border='light' background='white' className='mb-3' style={{ maxWidth: '18rem' }}>
<MDBCardHeader>Header</MDBCardHeader>
<MDBCardBody>
<MDBCardTitle>Light card title</MDBCardTitle>
<MDBCardText>
Some quick example text to build on the card title and make up the bulk of the card's content.
</MDBCardText>
</MDBCardBody>
</MDBCard>
<MDBCard shadow='0' border='dark' background='white' style={{ maxWidth: '18rem' }}>
<MDBCardHeader>Header</MDBCardHeader>
<MDBCardBody className='text-dark'>
<MDBCardTitle>Dark card title</MDBCardTitle>
<MDBCardText>
Some quick example text to build on the card title and make up the bulk of the card's content.
</MDBCardText>
</MDBCardBody>
</MDBCard>
</div>
);
}
Mixins utilities
You can also change the borders on the card header and footer as needed, and even remove
their background-color
with .bg-transparent
.
Success card title
Some quick example text to build on the card title and make up the bulk of the card's content.
import React from 'react';
import { MDBCard, MDBCardTitle, MDBCardText, MDBCardBody, MDBCardHeader, MDBCardFooter } from 'mdb-react-ui-kit';
export default function App() {
return (
<MDBCard border='success' background='white' shadow='0' className='mb-3' style={{ maxWidth: '18rem' }}>
<MDBCardHeader background='transparent' border='success'>
Header
</MDBCardHeader>
<MDBCardBody className='text-success'>
<MDBCardTitle>Success card title</MDBCardTitle>
<MDBCardText>
Some quick example text to build on the card title and make up the bulk of the card's content.
</MDBCardText>
</MDBCardBody>
<MDBCardFooter background='transparent' border='success'>
Footer
</MDBCardFooter>
</MDBCard>
);
}
Card layout
In addition to styling the content within cards, Bootstrap includes a few options for laying out series of cards. For the time being, these layout options are not yet responsive.
Card groups
Use card groups to render cards as a single, attached element with equal width and height
columns. Card groups start off stacked and use
display: flex;
to become attached with uniform dimensions starting at the
sm
breakpoint.
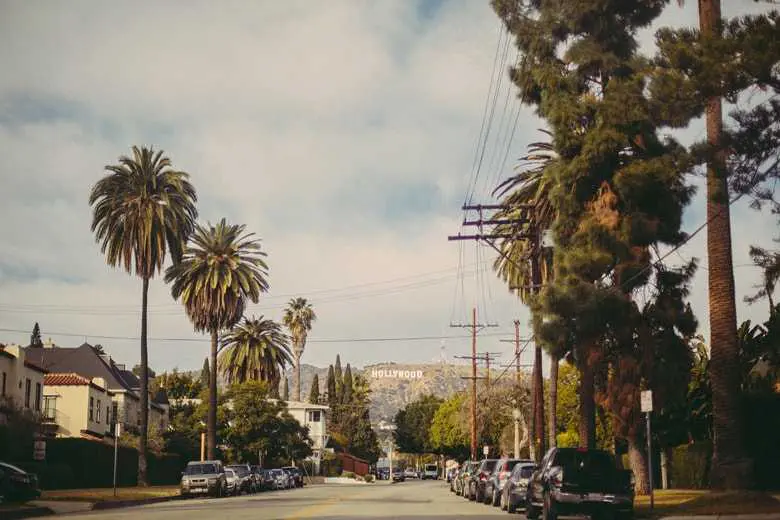
Card title
This is a wider card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.
Last updated 3 mins ago
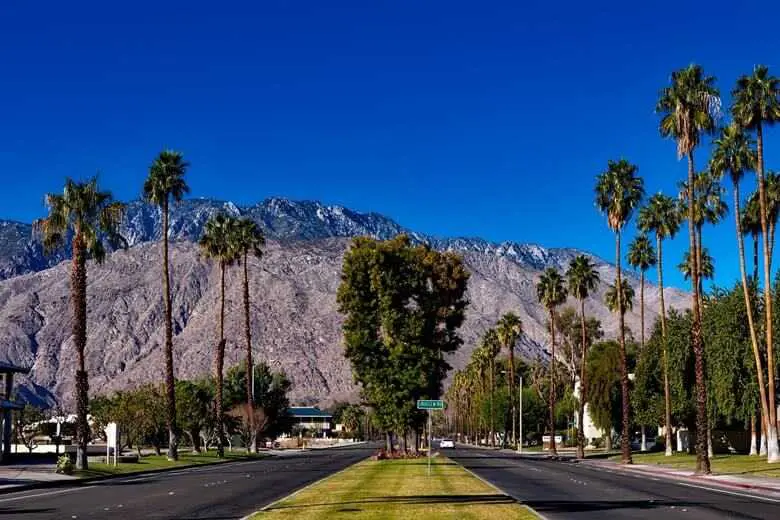
Card title
This card has supporting text below as a natural lead-in to additional content.
Last updated 3 mins ago
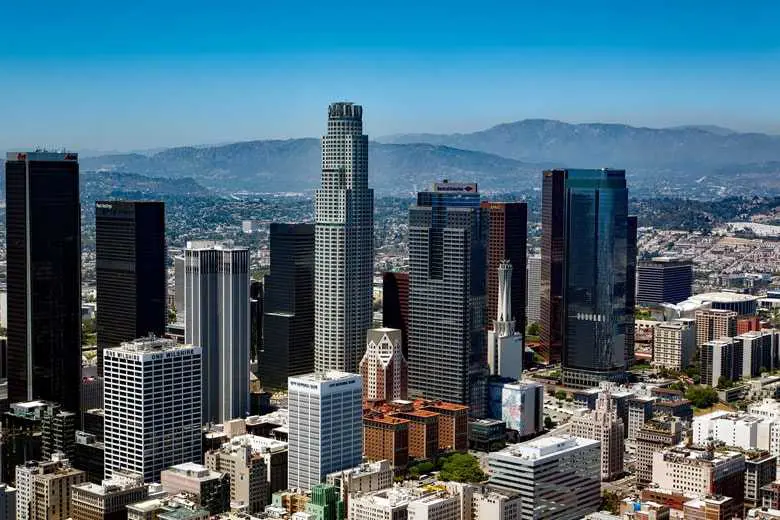
Card title
This is a wider card with supporting text below as a natural lead-in to additional content. This card has even longer content than the first to show that equal height action.
Last updated 3 mins ago
import React from 'react';
import { MDBCard, MDBCardImage, MDBCardBody, MDBCardTitle, MDBCardText, MDBCardGroup } from 'mdb-react-ui-kit';
export default function App() {
return (
<MDBCardGroup>
<MDBCard>
<MDBCardImage src='https://mdbootstrap.com/img/new/standard/city/041.webp' alt='...' position='top' />
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This is a wider card with supporting text below as a natural lead-in to additional content. This
content is a little bit longer.
</MDBCardText>
<MDBCardText>
<small className='text-muted'>Last updated 3 mins ago</small>
</MDBCardText>
</MDBCardBody>
</MDBCard>
<MDBCard>
<MDBCardImage src='https://mdbootstrap.com/img/new/standard/city/042.webp' alt='...' position='top' />
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This is a wider card with supporting text below as a natural lead-in to additional content.
</MDBCardText>
<MDBCardText>
<small className='text-muted'>Last updated 3 mins ago</small>
</MDBCardText>
</MDBCardBody>
</MDBCard>
<MDBCard>
<MDBCardImage src='https://mdbootstrap.com/img/new/standard/city/043.webp' alt='...' position='top' />
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This is a wider card with supporting text below as a natural lead-in to additional content. This
card has even longer content than the first to show that equal height action.
</MDBCardText>
<MDBCardText>
<small className='text-muted'>Last updated 3 mins ago</small>
</MDBCardText>
</MDBCardBody>
</MDBCard>
</MDBCardGroup>
);
}
When using card groups with footers, their content will automatically line up.
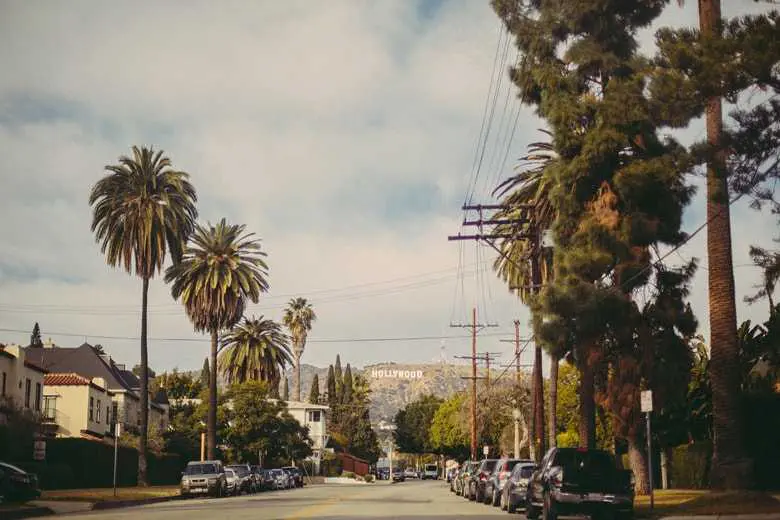
Card title
This is a wider card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.
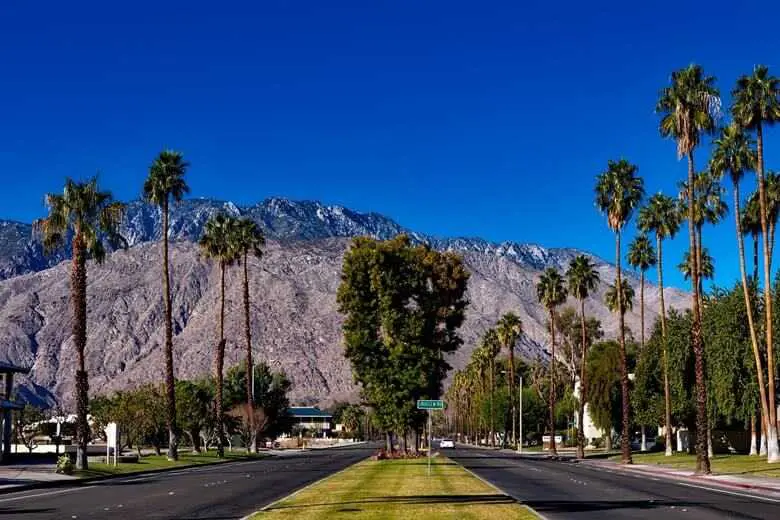
Card title
This card has supporting text below as a natural lead-in to additional content.
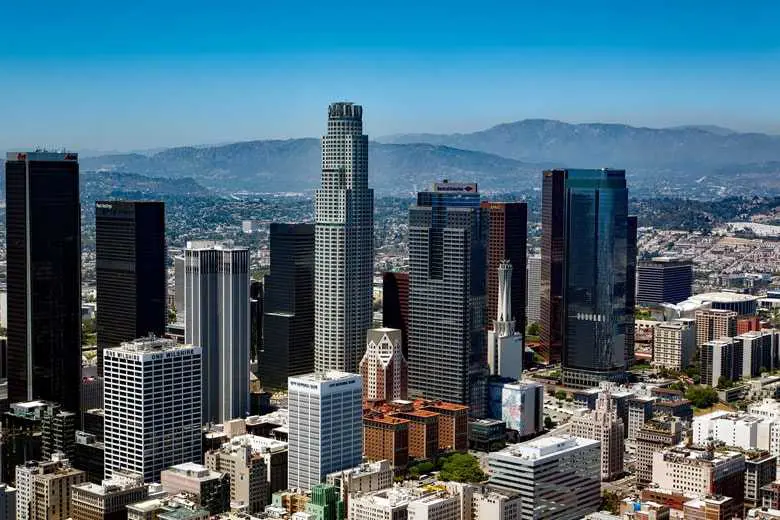
Card title
This is a wider card with supporting text below as a natural lead-in to additional content. This card has even longer content than the first to show that equal height action.
import React from 'react';
import { MDBCard, MDBCardImage, MDBCardBody, MDBCardTitle, MDBCardText, MDBCardFooter, MDBCardGroup } from 'mdb-react-ui-kit';
export default function App() {
return (
<MDBCardGroup>
<MDBCard>
<MDBCardImage src='https://mdbootstrap.com/img/new/standard/city/041.webp' alt='...' position='top' />
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This is a wider card with supporting text below as a natural lead-in to additional content. This
content is a little bit longer.
</MDBCardText>
</MDBCardBody>
<MDBCardFooter>
<small className='text-muted'>Last updated 3 mins ago</small>
</MDBCardFooter>
</MDBCard>
<MDBCard>
<MDBCardImage src='https://mdbootstrap.com/img/new/standard/city/042.webp' alt='...' position='top' />
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This is a wider card with supporting text below as a natural lead-in to additional content.
</MDBCardText>
</MDBCardBody>
<MDBCardFooter>
<small className='text-muted'>Last updated 3 mins ago</small>
</MDBCardFooter>
</MDBCard>
<MDBCard>
<MDBCardImage src='https://mdbootstrap.com/img/new/standard/city/043.webp' alt='...' position='top' />
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This is a wider card with supporting text below as a natural lead-in to additional content. This
card has even longer content than the first to show that equal height action.
</MDBCardText>
</MDBCardBody>
<MDBCardFooter>
<small className='text-muted'>Last updated 3 mins ago</small>
</MDBCardFooter>
</MDBCard>
</MDBCardGroup>
);
}
Grid cards
Use the Bootstrap grid system and its
.row-cols
classes to control how many grid columns (wrapped around your cards)
you show per row. For example, here’s .row-cols-1
laying out the cards on one
column, and .row-cols-md-2
splitting four cards to equal width across multiple
rows, from the medium breakpoint up.
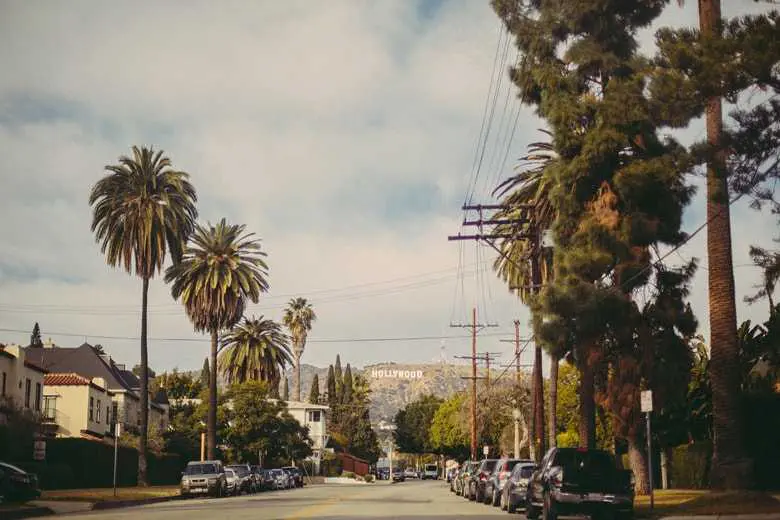
Card title
This is a longer card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.
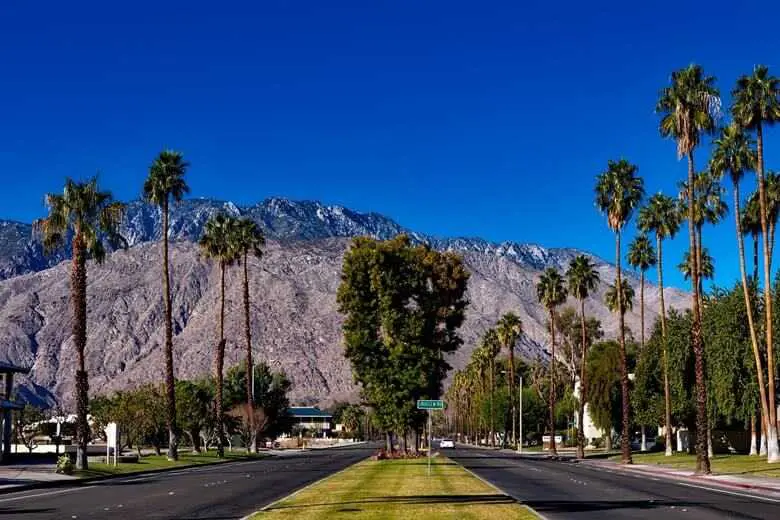
Card title
This is a longer card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.
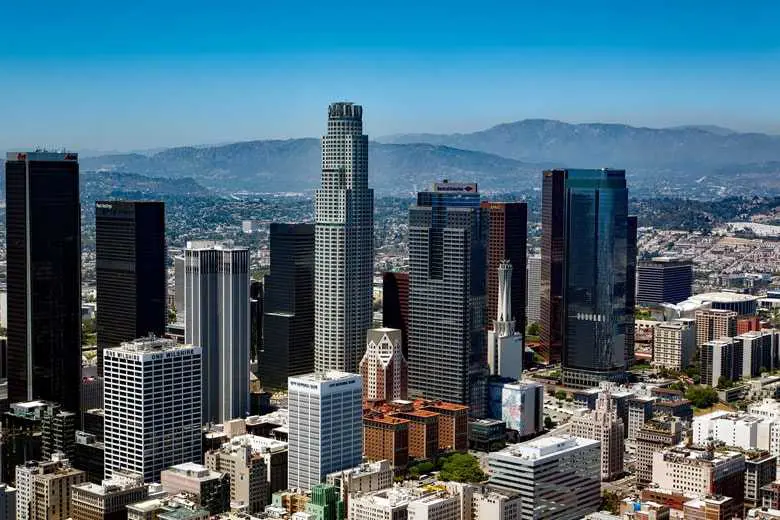
Card title
This is a longer card with supporting text below as a natural lead-in to additional content.
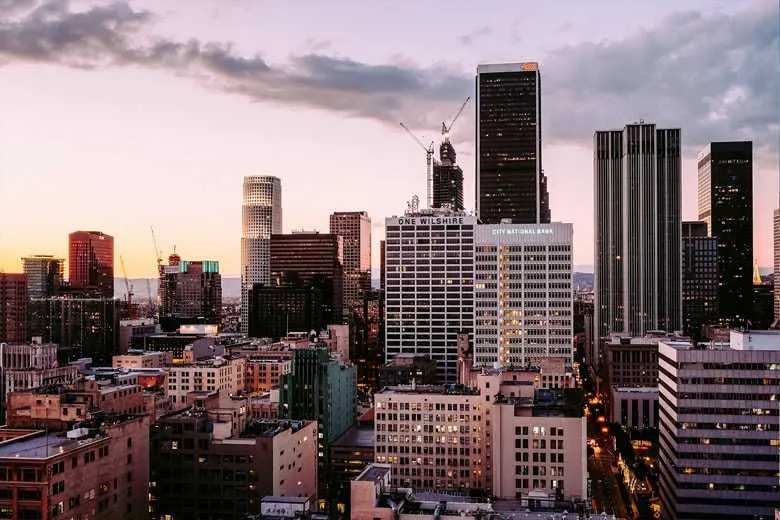
Card title
This is a longer card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.
import React from 'react';
import { MDBCard, MDBCardImage, MDBCardBody, MDBCardTitle, MDBCardText, MDBRow, MDBCol } from 'mdb-react-ui-kit';
export default function App() {
return (
<MDBRow className='row-cols-1 row-cols-md-2 g-4'>
<MDBCol>
<MDBCard>
<MDBCardImage
src='https://mdbootstrap.com/img/new/standard/city/041.webp'
alt='...'
position='top'
/>
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This is a longer card with supporting text below as a natural lead-in to additional content.
This content is a little bit longer.
</MDBCardText>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol>
<MDBCard>
<MDBCardImage
src='https://mdbootstrap.com/img/new/standard/city/042.webp'
alt='...'
position='top'
/>
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This is a longer card with supporting text below as a natural lead-in to additional content.
This content is a little bit longer.
</MDBCardText>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol>
<MDBCard>
<MDBCardImage
src='https://mdbootstrap.com/img/new/standard/city/043.webp'
alt='...'
position='top'
/>
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This is a longer card with supporting text below as a natural lead-in to additional content.
This content is a little bit longer.
</MDBCardText>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol>
<MDBCard>
<MDBCardImage
src='https://mdbootstrap.com/img/new/standard/city/044.webp'
alt='...'
position='top'
/>
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This is a longer card with supporting text below as a natural lead-in to additional content.
This content is a little bit longer.
</MDBCardText>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
);
}
Change it to .row-cols-3
and you’ll see the fourth card wrap.
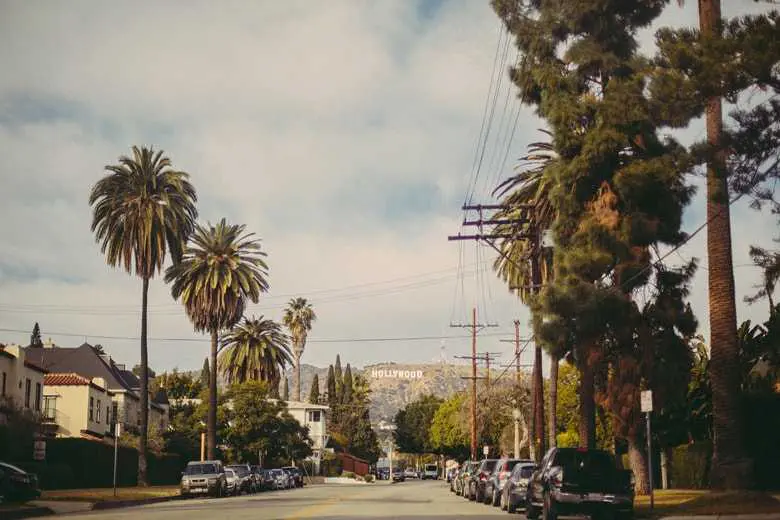
Card title
This is a longer card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.
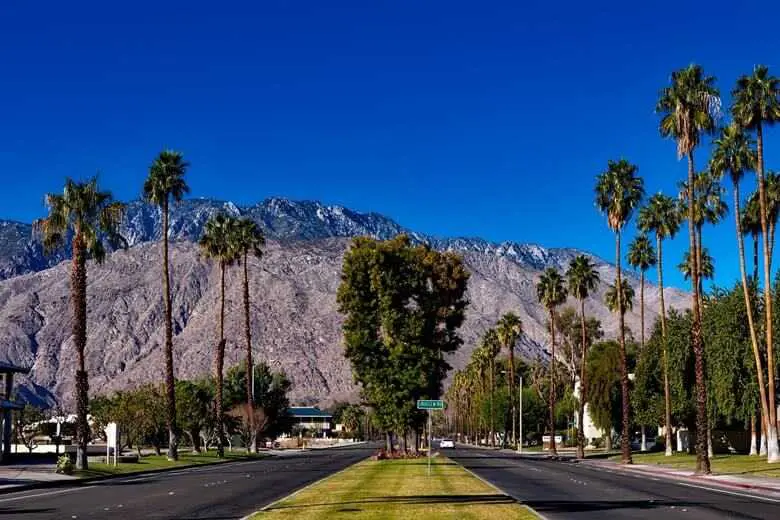
Card title
This is a longer card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.
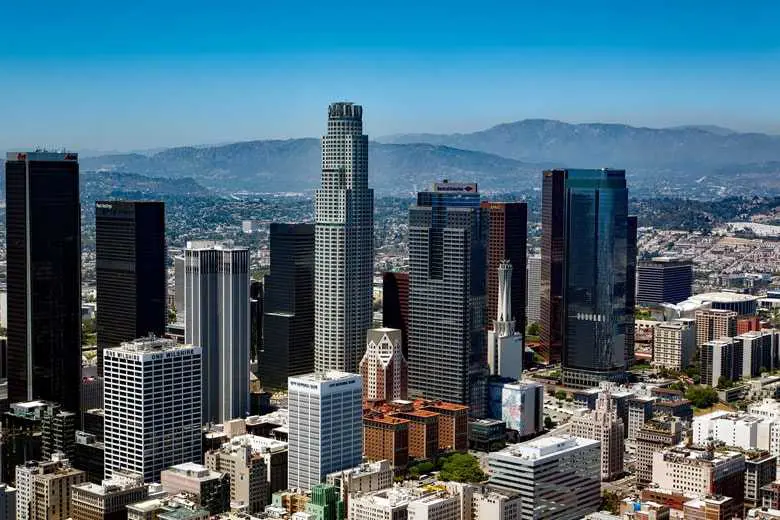
Card title
This is a longer card with supporting text below as a natural lead-in to additional content.
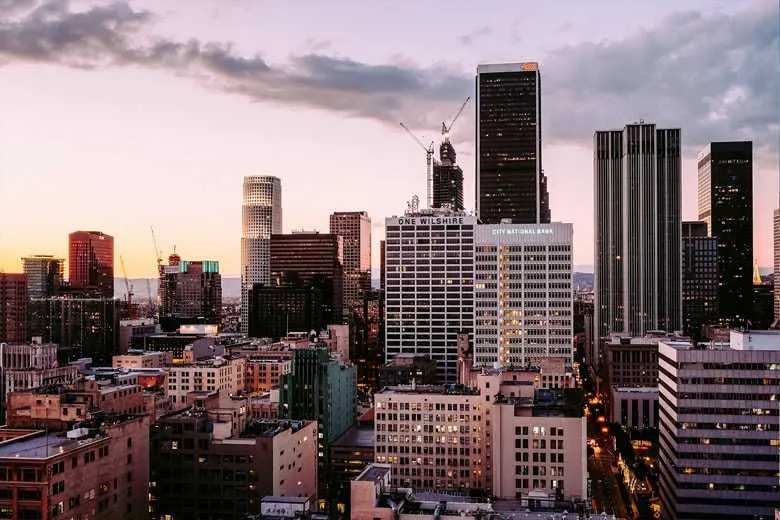
Card title
This is a longer card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.
import React from 'react';
import { MDBCard, MDBCardImage, MDBCardBody, MDBCardTitle, MDBCardText, MDBRow, MDBCol } from 'mdb-react-ui-kit';
export default function App() {
return (
<MDBRow className='row-cols-1 row-cols-md-3 g-4'>
<MDBCol>
<MDBCard>
<MDBCardImage
src='https://mdbootstrap.com/img/new/standard/city/041.webp'
alt='...'
position='top'
/>
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This is a longer card with supporting text below as a natural lead-in to additional content.
This content is a little bit longer.
</MDBCardText>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol>
<MDBCard>
<MDBCardImage
src='https://mdbootstrap.com/img/new/standard/city/042.webp'
alt='...'
position='top'
/>
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This is a longer card with supporting text below as a natural lead-in to additional content.
This content is a little bit longer.
</MDBCardText>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol>
<MDBCard>
<MDBCardImage
src='https://mdbootstrap.com/img/new/standard/city/043.webp'
alt='...'
position='top'
/>
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This is a longer card with supporting text below as a natural lead-in to additional content.
This content is a little bit longer.
</MDBCardText>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol>
<MDBCard>
<MDBCardImage
src='https://mdbootstrap.com/img/new/standard/city/044.webp'
alt='...'
position='top'
/>
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This is a longer card with supporting text below as a natural lead-in to additional content.
This content is a little bit longer.
</MDBCardText>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
);
}
When you need equal height, add .h-100
to the cards. If you want equal heights
by default, you can set $card-height: 100%
in Sass.
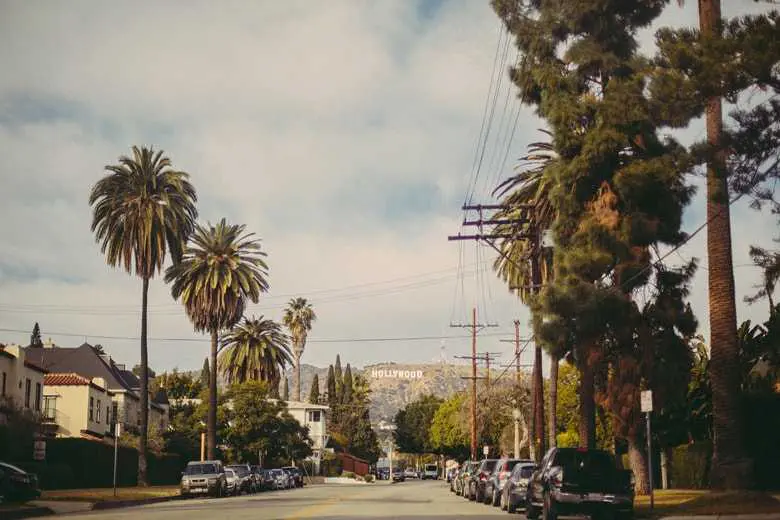
Card title
This is a longer card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.
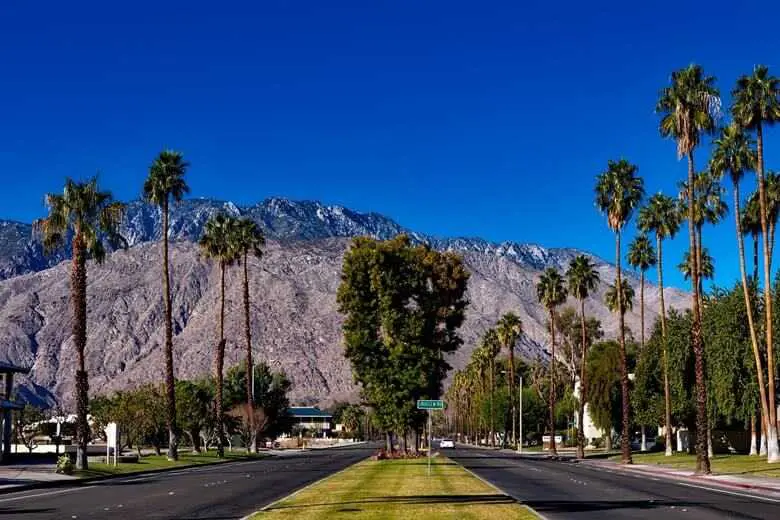
Card title
This is a short card.
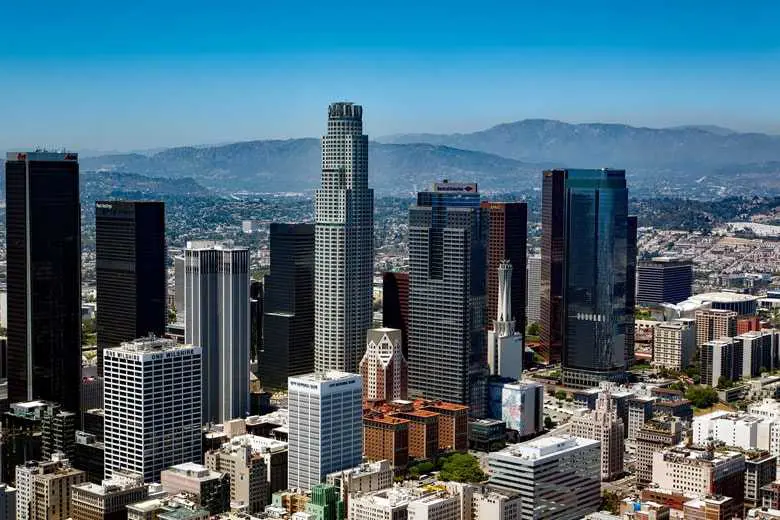
Card title
This is a longer card with supporting text below as a natural lead-in to additional content.
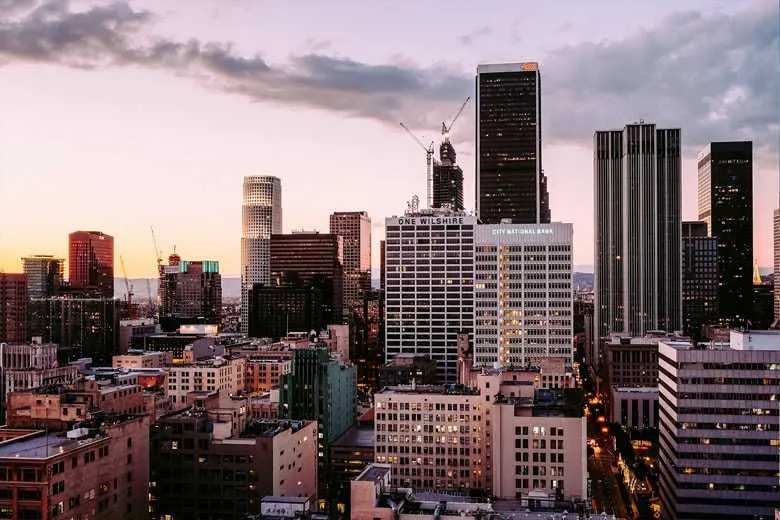
Card title
This is a longer card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.
import React from 'react';
import { MDBCard, MDBCardImage, MDBCardBody, MDBCardTitle, MDBCardText, MDBRow, MDBCol } from 'mdb-react-ui-kit';
export default function App() {
return (
<MDBRow className='row-cols-1 row-cols-md-3 g-4'>
<MDBCol>
<MDBCard className='h-100'>
<MDBCardImage
src='https://mdbootstrap.com/img/new/standard/city/041.webp'
alt='...'
position='top'
/>
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This is a longer card with supporting text below as a natural lead-in to additional content.
This content is a little bit longer.
</MDBCardText>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol>
<MDBCard className='h-100'>
<MDBCardImage
src='https://mdbootstrap.com/img/new/standard/city/042.webp'
alt='...'
position='top'
/>
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>This is a short card.</MDBCardText>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol>
<MDBCard className='h-100'>
<MDBCardImage
src='https://mdbootstrap.com/img/new/standard/city/043.webp'
alt='...'
position='top'
/>
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This is a longer card with supporting text below as a natural lead-in to additional content.
</MDBCardText>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol>
<MDBCard className='h-100'>
<MDBCardImage
src='https://mdbootstrap.com/img/new/standard/city/044.webp'
alt='...'
position='top'
/>
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This is a longer card with supporting text below as a natural lead-in to additional content.
This content is a little bit longer.
</MDBCardText>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
);
}
Just like with card groups, card footers will automatically line up.
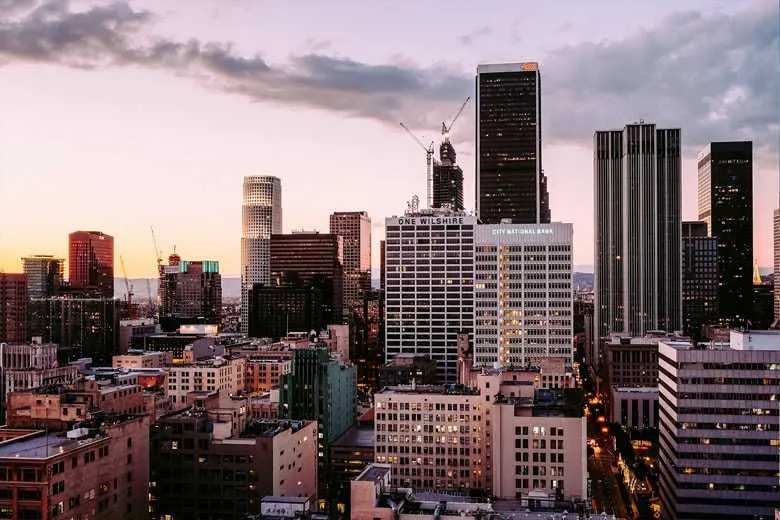
Card title
This is a wider card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.
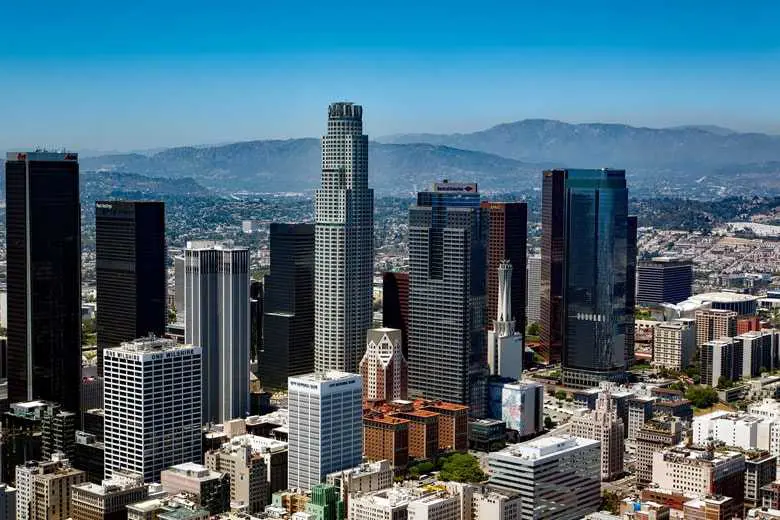
Card title
This card has supporting text below as a natural lead-in to additional content.
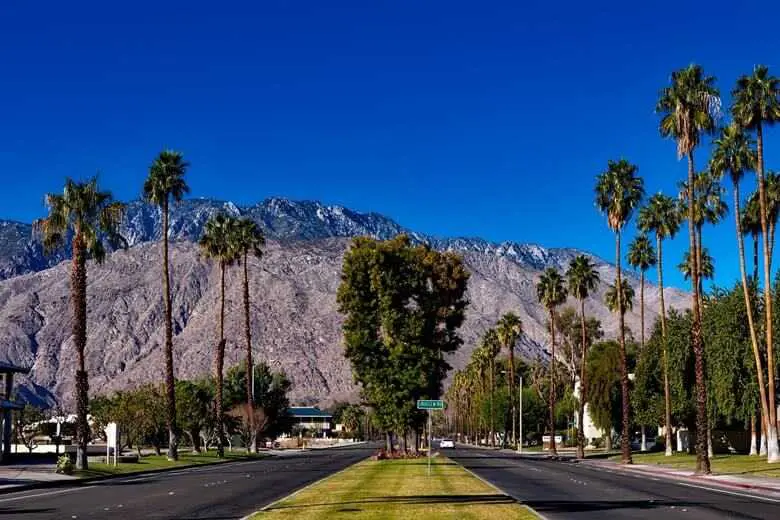
Card title
This is a wider card with supporting text below as a natural lead-in to additional content. This card has even longer content than the first to show that equal height action.
import React from 'react';
import { MDBCard, MDBCardImage, MDBCardBody, MDBCardTitle, MDBCardText, MDBCardFooter, MDBRow, MDBCol } from 'mdb-react-ui-kit';
export default function App() {
return (
<MDBRow className='row-cols-1 row-cols-md-3 g-4'>
<MDBCol>
<MDBCard className='h-100'>
<MDBCardImage
src='https://mdbootstrap.com/img/new/standard/city/044.webp'
alt='...'
position='top'
/>
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This is a longer card with supporting text below as a natural lead-in to additional content.
This content is a little bit longer.
</MDBCardText>
</MDBCardBody>
<MDBCardFooter>
<small className='text-muted'>Last updated 3 mins ago</small>
</MDBCardFooter>
</MDBCard>
</MDBCol>
<MDBCol>
<MDBCard className='h-100'>
<MDBCardImage
src='https://mdbootstrap.com/img/new/standard/city/043.webp'
alt='...'
position='top'
/>
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This card has supporting text below as a natural lead-in to additional content.
</MDBCardText>
</MDBCardBody>
<MDBCardFooter>
<small className='text-muted'>Last updated 3 mins ago</small>
</MDBCardFooter>
</MDBCard>
</MDBCol>
<MDBCol>
<MDBCard className='h-100'>
<MDBCardImage
src='https://mdbootstrap.com/img/new/standard/city/042.webp'
alt='...'
position='top'
/>
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
This is a wider card with supporting text below as a natural lead-in to additional content. This
card has even longer content than the first to show that equal height action.
</MDBCardText>
</MDBCardBody>
<MDBCardFooter>
<small className='text-muted'>Last updated 3 mins ago</small>
</MDBCardFooter>
</MDBCard>
</MDBCol>
</MDBRow>
);
}
Cards - API
Import
import {
MDBCard,
MDBCardBody,
MDBCardFooter,
MDBCardHeader,
MDBCardImage,
MDBCardOverlay,
MDBCardSubTitle,
MDBCardTitle,
MDBCardText,
MDBCardGroup,
MDBCardLink,
} from 'mdb-react-ui-kit';
Properties
MDBCard
Name | Type | Default | Description | Example |
---|---|---|---|---|
tag
|
String | 'div' |
Defines tag of the MDBCard element |
<MDBCard tag="section" />
|
className
|
String | '' |
Add custom class to MDBCard |
<MDBCard className="class" />
|
border
|
String | '' |
Sets a border for a card |
<MDBCard border="secondary" />
|
shadow
|
String | '' |
Sets a shadow for a card |
<MDBCard shadow="1" />
|
background
|
String | '' |
Sets a background color for a card |
<MDBCard background="secondary" />
|
alignment
|
String | '' |
Defines a text alignment inside the card |
<MDBCard alignment="end" />
|
MDBCardHeader
Name | Type | Default | Description | Example |
---|---|---|---|---|
tag
|
String | 'div' |
Defines tag of the MDBCardHeader element |
<MDBCardHeader tag="section" />
|
className
|
String | '' |
Add custom class to MDBCardHeader |
<MDBCardHeader className="class" />
|
border
|
String | '' |
Sets a border for a MDBCardHeader |
<MDBCardHeader border="secondary" />
|
background
|
String | '' |
Sets a background color for a MDBCardHeader |
<MDBCardHeader background="secondary" />
|
MDBCardBody
Name | Type | Default | Description | Example |
---|---|---|---|---|
tag
|
String | 'div' |
Defines tag of the MDBCardBody element |
<MDBCardBody tag="section" />
|
className
|
String | '' |
Add custom class to MDBCardBody |
<MDBCardBody className="class" />
|
MDBCardGroup
Name | Type | Default | Description | Example |
---|---|---|---|---|
tag
|
String | 'div' |
Defines tag of the MDBCardGroup element |
<MDBCardGroup tag="section" />
|
className
|
String | '' |
Add custom class to MDBCardGroup |
<MDBCardGroup className="class" />
|
MDBCardLink
Name | Type | Default | Description | Example |
---|---|---|---|---|
className
|
String | '' |
Add custom class to MDBCardLink |
<MDBCardLink className="class" />
|
MDBCardOverlay
Name | Type | Default | Description | Example |
---|---|---|---|---|
tag
|
String | 'div' |
Defines tag of the MDBCardOverlay element |
<MDBCardOverlay tag="section" />
|
className
|
String | '' |
Add custom class to MDBCardOverlay |
<MDBCardOverlay className="class" />
|
MDBCardTitle
Name | Type | Default | Description | Example |
---|---|---|---|---|
tag
|
String | 'h5' |
Defines tag of the MDBCardTitle element |
<MDBCardTitle tag="section" />
|
className
|
String | '' |
Add custom class to MDBCardTitle |
<MDBCardTitle className="class" />
|
MDBCardSubTitle
Name | Type | Default | Description | Example |
---|---|---|---|---|
tag
|
String | 'p' |
Defines tag of the MDBCardSubTitle element |
<MDBCardSubTitle tag="section" />
|
className
|
String | '' |
Add custom class to MDBCardSubTitle |
<MDBCardSubTitle className="class" />
|
MDBCardText
Name | Type | Default | Description | Example |
---|---|---|---|---|
tag
|
String | 'p' |
Defines tag of the MDBCardText element |
<MDBCardText tag="section" />
|
className
|
String | '' |
Add custom class to MDBCardText |
<MDBCardText className="class" />
|
MDBCardImage
Name | Type | Default | Description | Example |
---|---|---|---|---|
className
|
String | '' |
Add custom class to MDBCardImage |
<MDBCardImage className="class" />
|
overlay
|
Boolean | '' |
Adds a .card-img className for overlay purposes
|
<MDBCardImage overlay />
|
fluid
|
Boolean | '' |
Adds a .img-fluid className |
<MDBCardImage fluid />
|
position
|
String | '' |
Sets an image position in the card |
<MDBCardImage position="top" />
|